A Kubernetes pod serves as an isolated environment for running a single process, representing the smallest operational unit within the Kubernetes ecosystem. Unlike other resources, such as Deployments, which are replaced during updates, pods have a more persistent nature. Consequently, Kubernetes does not provide a dedicated kubectl restart pod
command. Instead, various methods, both automatic and manual, can be employed to restart pods effectively.
The short answer
To restart a Kubernetes pod, you can use the kubectl delete pod command as follows:
$ kubectl delete pod <pod_name>
Where:
- pod_name is the name of the pod to delete
Kubernetes will delete the pod and automatically recreate a new one with the same configuration.
Easily retrieve this command using Warp’s AI Command Suggestions
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Suggestions feature:
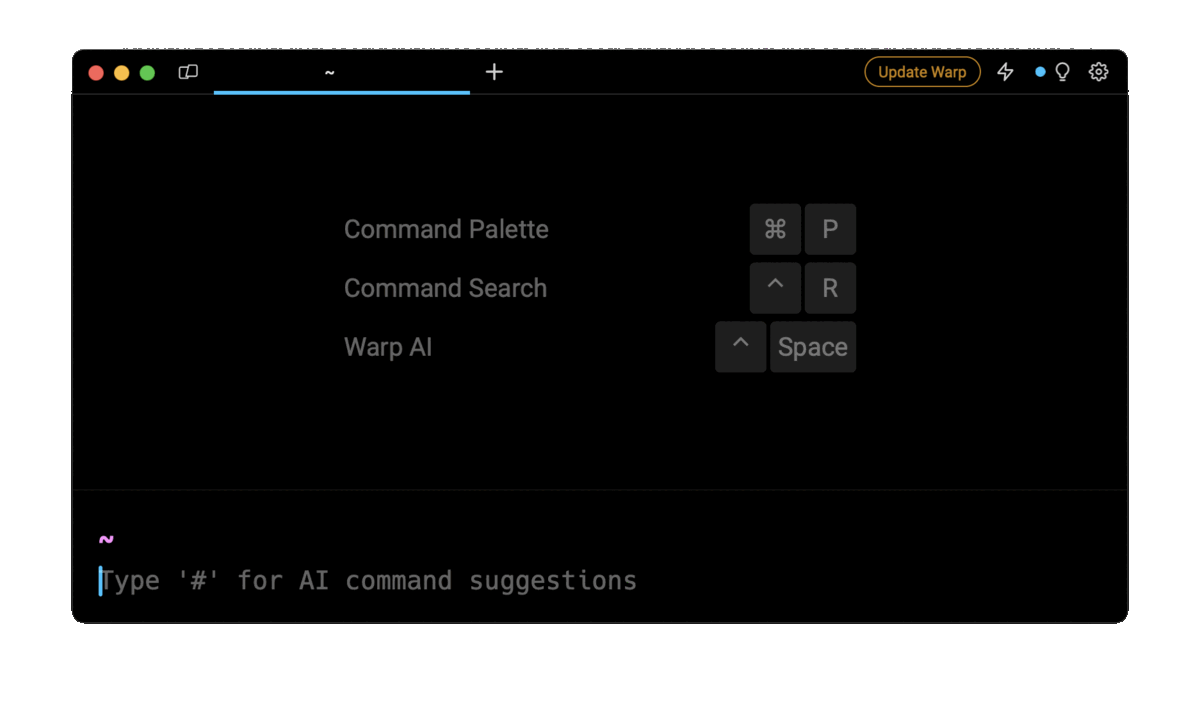
Entering kubectl delete pod in the AI Command Suggestions will prompt a kubectl command that can then quickly be inserted into your shell by doing CMD+ENTER.
Deleting multiple pods
To delete all the pods that have failed across all namespaces, you can use the following command:
$ kubectl delete pods --field-selector status.phase=Failed -A
Where:
- The --field-selector flag with status.phase=Failed specifies failed pods.
- The -A flag (short for --all-namespaces) specifies all the namespaces in the cluster.
Deleting pods in a namespace
To delete all the failed pods in a particular namespace, you can use the following command instead:
$ kubectl delete pods --field-selector status.phase=Failed --namespace=<namespace>
Where:
- namespace is the name of the namespace from which to delete the failed pods.
Deleting pods by label
If you’ve used labels to organize your pods, you can delete pods with a specific label using the -l flag as follows:
$ kubectl delete pods -l <label>=<value>
For example:
$ kubectl delete pods -l environment=test
The above command will delete all the pods with the environment label set to test.
Defining a restart policy
Pod specifications include a restartPolicy field used to define the restart policy associated with a pod. The available values are Always, OnFailure, and Never.
The Always restart policy
Always is the default restart policy. It ensures that Kubernetes will automatically restart a container within the pod whenever it terminates, whether it exits successfully or fails. As in the example below, a web server is a common use case for a restart policy of Always.
apiVersion: v1
kind: Pod
metadata:
name: nginx-webserver-pod
spec:
restartPolicy: Always
containers:
- name: my-container
image: nginx:latest
The OnFailure restart policy
The OnFailure restart policy is used for pods whose containers execute tasks on a schedule or only once. This ensures that the containers in the pod are replaced automatically in the event of a failure and prevents them from restarting unnecessarily, thus consuming resources.
The example below of a container that copies a file from one directory to another is a use case for setting the restart policy to OnFailure.
apiVersion: v1
kind: Pod
metadata:
name: busybox-file-task
spec:
restartPolicy: OnFailure
containers:
- name: busybox-container
image: busybox:latest
command: ["cp", "/path/to/source/file", "/path/to/destination/file"]
Restarting pods using rolling updates
Aside from choosing the appropriate restart policy, there are many scenarios where it becomes necessary to restart a pod manually. The most recommended method for a manual restart is the rolling restart.
A rollout is a process used to ensure that updates are rolled out with minimal disruption of the availability of the application by terminating pods gracefully and recreating them one at a time. This method is available in Kubernetes v1.15 and later.
Performing the rolling restart of a Deployment
A Deployment controller can be defined to declare the desired application state, including details such as the container image, number of replicas, and update strategy. A common use case is managing the deployment and scaling of a web application.
If a change is made to the Deployment manifest, all the pods in the Deployment can be restarted with the command below:
$ kubectl rollout restart deployment/<deployment_name>
Performing a rolling restart using namespaces
Namespaces are logical, isolated environments within a Kubernetes cluster that allow you to group and manage Kubernetes resources separately.
To restart all the Deployments located in a specified namespace, you can use the following kubectl rollout restart deployment command with the -n flag as follows:
$ kubectl rollout restart deployment/<deployment_name>-n<namespace>
Performing a rolling restart using labels
Labels are key-value pairs attached to Kubernetes objects used for organizing resources based on specific criteria.
To restart all the pods based on a specific label, you can use the kubectl rollout restart pod command with the -l flag as follows:
$ kubectl rollout restart pod -l <label>=<value>
For example:
$ kubectl rollout restart pod -l environment=development
The above command will restart all the pods identified by the environment=development label.
Scaling down deployment replicas
Scaling pods down is another method to restart pods in a controlled manner with graceful termination.
To restart a pod by scaling deployment replicas to 0, you can use the following kubectl scale deployment command:
$ kubectl scale --replicas=0 deployment <deployment_name>
Which will gracefully terminate all the pods in the specified deployment.
You can then recreate them by setting the number of replicas to 1 or more as follows:
$ kubectl scale --replicas=1 deployment <deployment_name>
Scaling down Deployments in a namespace
To restart the pods in a particular namespace, you can use the kubectl scale deployment with the -n flag as follows:
$ kubectl scale --replicas=0 deployment <deployment_name> -n <namespace>
$ kubectl scale --replicas=1 deployment <deployment_name> -n <namespace>
Scaling down Deployment using labels
To restart pods with a specific label, you can use the kubectl scale command with the --selector flag as follows:
$ kubectl scale --replicas=0 --selector=<label>=<value> deployment
$ kubectl scale --replicas=1 --selector=<label>=<value> deployment
Where:
- label is the name of the label selector.
- value is the value associated with this label.
For example:
$ kubectl scale --replicas=0 --selector=app=my-app deployment
$ kubectl scale --replicas=1 --selector=app=my-app deployment
Updating environment variables
Updating the environment variables of a pod, or container in a pod, will gracefully terminate the pod, change the specified environment variables, and recreate the pod.
Updating the environment variables of a pod
To update the environment variables of a pod, you can use the kubectl set env command as follows:
$ kubectl set env pod <pod_name> -n<namespace> <env_name> =<env_value>
Where:
- pod_name is the name of the pod you want to update.
- namespace is the name of the namespace the pod runs in.
- env_name is the name of the environment variable you want to add or update.
- env_value is the value of the
env\_name
variable.
For example:
$ kubectl set env pod my-pod -n my-namespace USER_NAME=admin USER_PWD=admin
Updating the environment variables of a container
To update the environment variables of a specific container in a pod, you can use the kubectl set env command as follows:
$ kubectl set env pod <pod_name> -n <namespace> -c <container_name> <env_name>=<env_value>
Where:
- pod_name is the name of the pod you want to update.
- namespace is the name of the namespace the pod runs in.
- container_name is the name of the container in the pod.
- env_name is the name of the environment variable you want to add or update.
- env_value is the value of the env_name variable.
For example:
$ kubectl set env pod my-pod -n my-namespace -c database DB_NAME=admin DB_PWD=admin
Written by
Sudha Bulusu
Filed Under
Related Articles
Copy Files From Pod in Kubernetes
Learn how to copy files and directories from within a Kubernetes Pod into the local filesystem using the kubectl command.
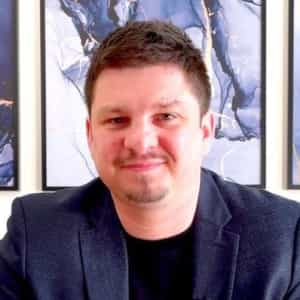
Scale Deployments in Kubernetes
Learn how to manually and automatically scale a Deployment based on CPU usage in Kubernetes using the kubectl-scale and kubectl-autoscale commands.
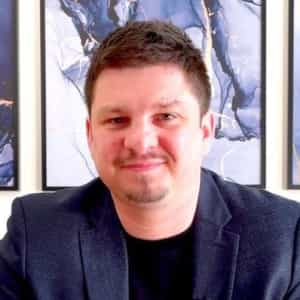
Get Kubernetes Logs With kubectl
Learn how to get the logs of pods, containers, deployments, and services in Kubernetes using the kubectl command. Troubleshoot a cluster stuck in CrashloopBackoff, ImagePullBackoff, or Pending error states.
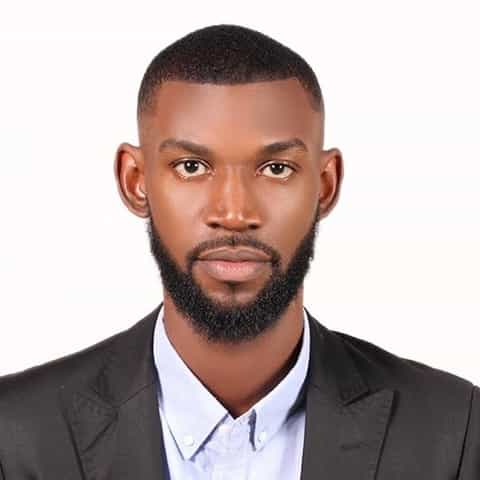
Forward Ports In Kubernetes
Learn how to forward the ports of Kubernetes resources such as Pods and Services using the kubectl port-forward command.
Tail Logs In Kubernetes
Learn how to tail and monitor Kubernetes logs efficiently to debug, trace, and troubleshoot errors more easily using the kubectl command.
Get Context In Kubernetes
Learn how to get information about one or more contexts in Kubernetes using the kubectl command.
Delete Kubernetes Namespaces With kubectl
Learn how to delete one or more namespaces and their related resources in a Kubernetes cluster using the kubectl command.
Get Kubernetes Secrets With kubectl
Learn how to list, describe, customize, sort and filter secrets in a Kubernetes cluster by name, type, namespace, label and more using the kubectl command.
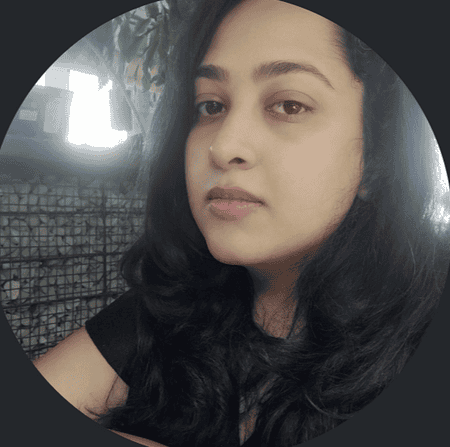
List Kubernetes Namespaces With kubectl
Learn how to list, describe, customize, sort and filter namespaces in a Kubernetes cluster by name, label, and more using the kubectl command.
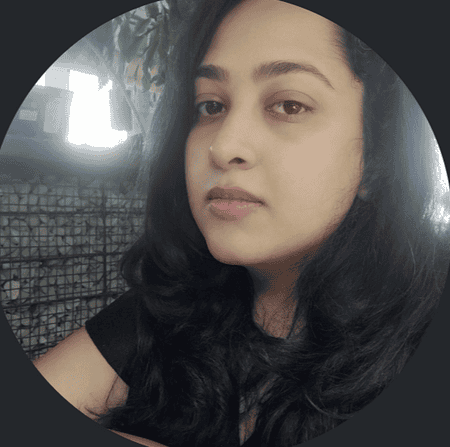
How To List Events With kubectl
Learn how to list and filter events in Kubernetes cluster by namespace, pod name and more using the kubectl command.
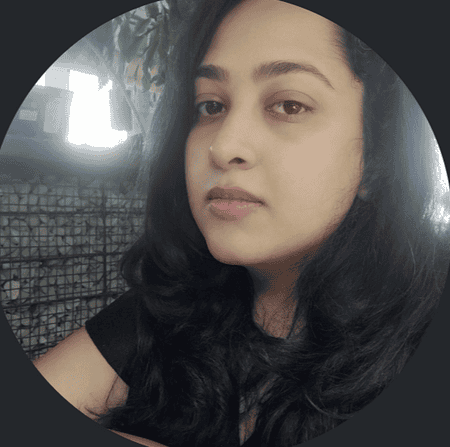
Kubernetes vs Docker: The Backbone of Modern Backend Technologies
Lean the fundamentals of the Kubernetes and Docker technologies and how they interplay with each other.
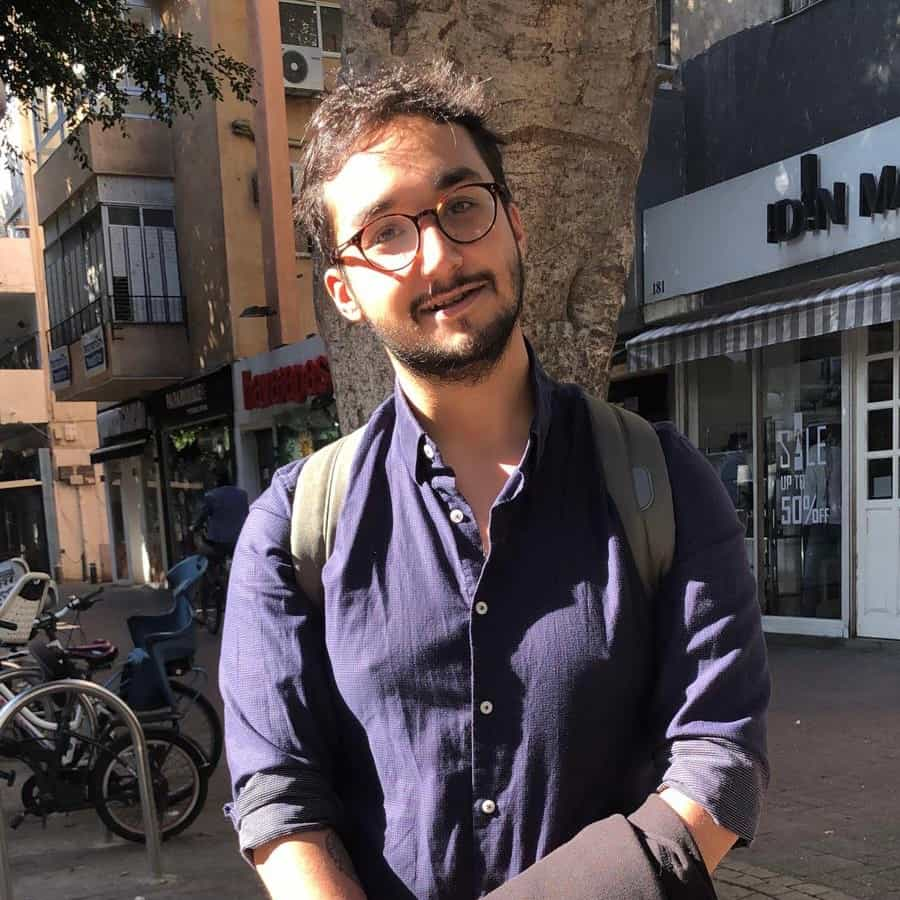
Set Context With kubectl
Learn how to create, modify, switch, and delete a context in Kubernetes using the kubectl config command.
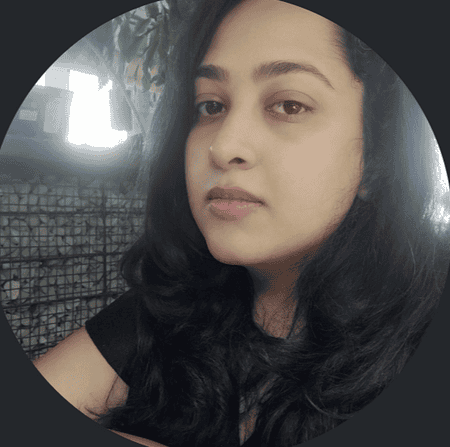