There are two approaches to deleting a file in Python:
- 1. Using Python’s built-in OS Module (Recommended)
- 2. Using the Pathlib Module.
Python's built-in OS module provides functions to interact with the operating system. You can use it to create and delete files/directories, access their contents, change directories, and more.
The Pathlib module was introduced in Python 3.4 and above (it can be installed using pip for prior versions). Pathlib makes it easier for users to interact with file paths and creates path objects. Pathlib Module is said to be more efficient in certain tasks (ex:. single paths, making things, and reading + finding things). However, when it comes to the task of removing things, both modules work equally as well. So feel free to use whichever approach you feel more comfortable with.
Using the OS Module (Recommended)
Here's the python code to delete a file using os.remove:
import os
file_path = '/tmp/file.txt'
os.remove(file_path)
The code above imports the os module, and then deletes the file named file.txt
If you would like to try it out, create a new python file:
touch myPythonFile.py
Let's open the file we just created:
nano myPythonFile.py
paste the following code:
import os
file_path = '/tmp/file.txt'
os.remove(file_path)
Run the python file with python myPythonFile.py This will delete the file mentioned in the filepath.
The OS module provides two methods to delete a file - os.remove(file_path) and os.unlink(). Both of them perform the same function. As stated in the Python OS Documentation, the only reason there are two versions of the identical method is because .unlink() is the traditional name in Unix.
Pathlib Module
Here's the python code to delete a file using file_pathlib.unlink():
from pathlib import Path
file_path = Path('/tmp/file.txt')
file_path.unlink()
The code above imports the pathlib module, and then deletes the file named file.txt
If you would like to try it out, create a new python file:
touch myPythonFile.py
Let's open the file we just created:
nano myPythonFile.py
paste the following code:
from pathlib import Path
file_path = Path('/tmp/file.txt')
file_path.unlink()" > myPythonfile.py
Run the python file with python myPythonFile.py This will delete the file mentioned in the filepath.
Errors That Could Arise and Their Remedies
IsADirectoryError
Both os.remove() and os.unlink() support deleting only files, not directories. An IsADirectoryError will be raised if you try to delete a directory.
FileNotFoundError
A ‘FileNotFoundError’ error will be raised if you attempt to delete a file that does not exist.
PermissionError
Deleting a file requires writing and executing a permission on its parent directory. A PermissionError is raised if you try to delete a file that does have the correct permissions set on the parent directory.
It's good practice to handle these error exceptions in your code by using try, except statements.
Check if a file exists with OS Module:
import os
file_path = '/tmp/file.txt'
try:
os.remove(file_path)
except OSError as e:
print("Error: %s : %s" % (file_path, e.strerror))
Check if a file exists with Pathlib:
from pathlib import Path
file_path = Path('/tmp/file.txt')
try:
file_path.unlink()
except OSError as e:
print("Error: %s : %s" % (file_path, e.strerror))
Written by
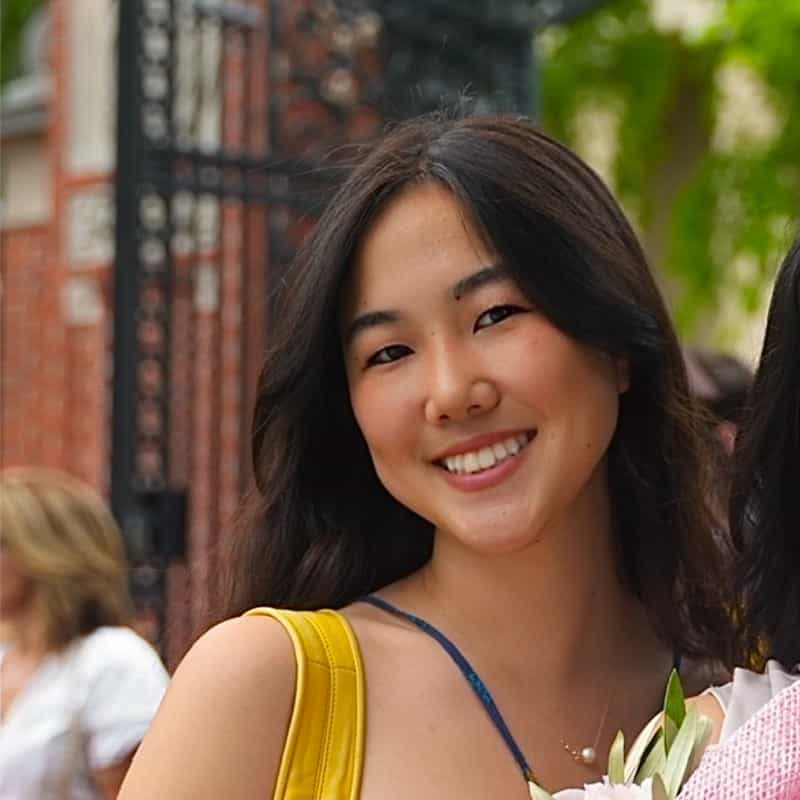
Rachel Hong
CS, Berkeley
Filed Under