Execute Commands in Pods With kubectl
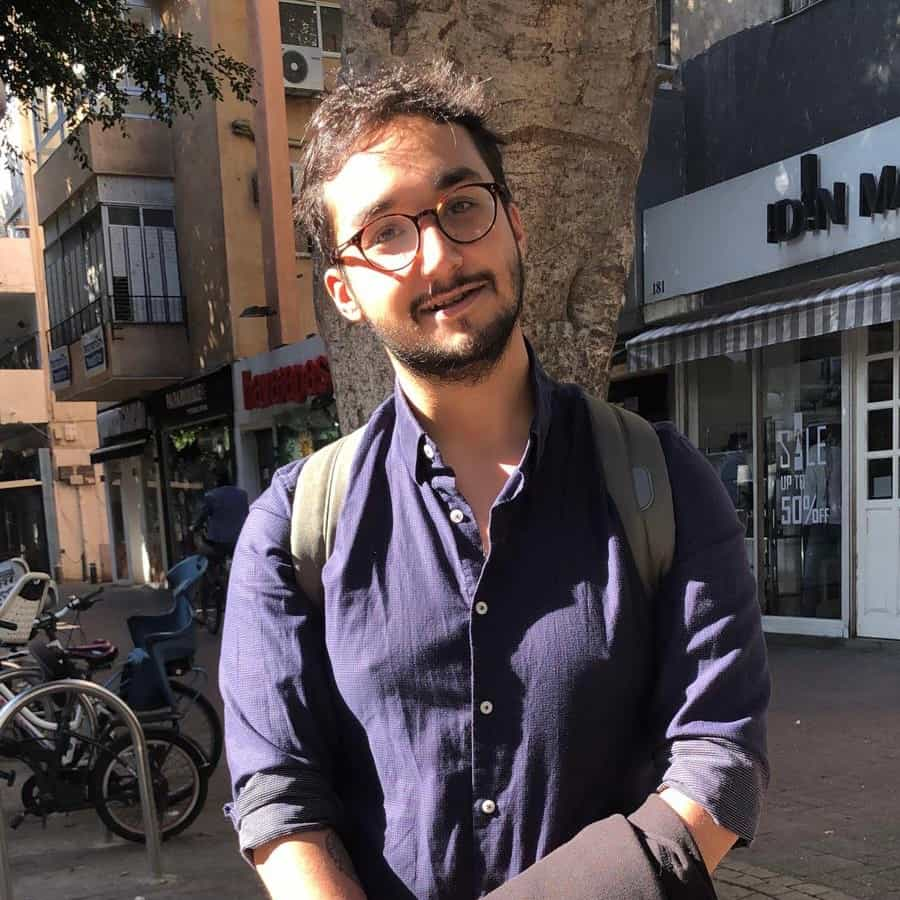
Gabriel Manricks
Chief Architect, ClearX
Published: 8/13/2024
The short answer
In Kubernetes, to execute a command inside a running Pod’s container, you can use the kubectl exec command as follows:
$ kubectl exec <pod> -- <command>
Where:
- pod is the name of the pod you want to execute a command into.
- command is the command you want to execute.
- -- the double dash separates the kubectl parameters and flags from the command you want to run and its flags/parameters.
For example:
$ kubectl exec web-0 -- env
This will execute the env command within a Pod named web-0, resulting in its environment variables being outputted to the terminal window.
Easily retrieve this command using Warp’s AI Command Suggestions
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Suggestions feature:
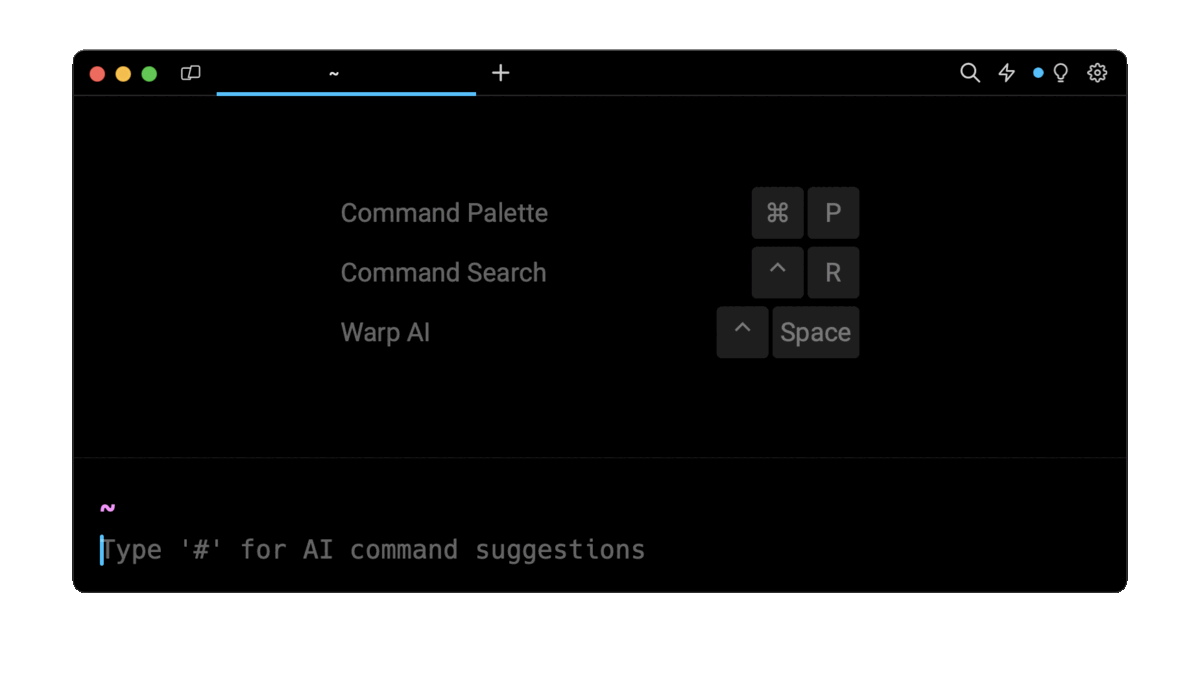
Pressing the # symbol at the start of a line will pull up the AI search and then you can enter kubernetes execute command to get the command template. You can quickly insert that command into your shell using CMD+ENTER.
Handling the “command / executable file not found” error
When trying to execute a command within a Pod, you need to make sure that this command is defined in the PATH environment variable of the container, as it might otherwise result in an “executable not found” error. In that case, you can use the full path to the executable.
For example:
$ kubectl exec web-0 -- /opt/redis-cli --help
This command will execute the redis-cli utility located in the /opt directory of the Pod named web-0.
Running interactive commands
By default kubectl exec will run the specified command without being attached to the standard input, which means that the command will run and immediately exit.
If you need to run a program that requires user input, you can run the kubectl exec command using the -i and -t flags:
$ kubectl exec -i -t <pod name> -- <command>
Where:
- -i connects your terminal to the standard input of the command’s process.
- -t wraps the connection in a pseudo-TTY providing an enhanced terminal-like interface.
For example, to launch the NodeJS runtime in a Pod with the node command, you can run the following kubectl command:
$ kubectl exec -it nodejs-pod -- node
This works exactly like running interactive commands in Docker, which you can learn more about by reading our article on how to run a Bash shell in Docker.
Starting an interactive shell session in a Pod's container
Often, it’s useful to start an interactive shell session instead of running a one-off command, for situations where you need to perform multiple manual actions or debug a container.
Most of the time you will want to simply run bash, as it has pretty good odds of being defined in the PATH environment variable and provides a better experience then the sh shell.
To start an interactive shell session with Bash, you can use the following command:
$ kubectl exec -it <pod> -- bash
Finding the list of available shells
If the bash command is not directly available in the PATH of the container, you can try one of the following popular shell paths instead:
- /bin/bash
- /bin/sh
- /bin/zsh
- /bin/ash
If none of these paths work, you may want to perform a search in folders like /usr/bin, which can be automated using the following command:
$ kubectl exec web-0 -- find /bin /usr/bin -name "*sh"
This command will search in the /bin and /usr/bin for anything ending with sh. Some of these might not be shells, but most of the shells end with "sh". If you don’t have find you can use ls to manually go through them (combined with a local grep) to get a similar result.
Executing a command in a Pod with multiple containers
By default, kubectl exec will execute the specified command in the first container listed in the YAML Pod spec file.
To execute a command in a specific container instead, you can use the -c flag followed by the container’s name:
$ kubectl exec -it <pod> -c <container> -- <command>
For example:
$ kubectl exec -it web-0 -c monitor -- bash
This command will execute the bash command within the container named monitor, that runs within the Pod named web-0.
Note that to discover the names of the containers running within a Pod, you can either manually inspect the Pod's YAML file or use the kubectl get pods command as follows:
$ kubectl get pods web-0 -o jsonpath='{.spec.containers[*].name}'
Executing a command in a Pod via a connected Kubernetes resource
By default, the kubectl exec command assumes that the specified resource a command should be executed on is a Pod.
To execute a command on another resource, such as a Deployment, a Statefulset or a Service, you can use the following syntax:
$ kubectl exec [-it] <resource>/<name> -- <command>
Where:
- resource is the type of the resource (e.g. deployment, service).
- name is the name of the resource.
- command is the command to execute on the Pod managed by that resource.
For example:
$ kubectl exec -it deployment/nginx -- bash
This command will execute the bash utility in interactive mode in the first container of the first Pod managed by the nginx Deployment.
Executing a command in a Pod using a YAML manifest
Another way to execute a command in a Pod is to use the YAML manifest the Pod or the Deployment was created from.
To achieve this, you can use the -f flag (short for --filename) as follows:
$ kubectl exec -f <file> -- <command>
Where:
- file is the relative or absolute path to the YAML manifest file.
- command is the command to execute on the Pod declared in this file.
For example:
$ kubectl exec -f deployment.yaml -- ls /
This command will read the deployment.yaml file and execute the ls / command in the first Pod of the replicaset of the Deployment specified in that file.
Note that, if the YAML manifest contains more than one Deployment / Pod, the kubectl exec command will fail.
Handling limited user privileges
When running a command using kubectl exec, the command will run using the same user that the container is running as. In Kubernetes (and Docker) you can specify any number as the user ID and the container will run as that user, even if no user was ever created with the ID. This is often used as a security feature where Pods will run using one of these non-existing users (typically User ID 1000/1001) providing very little access inside the container.
Sometimes you require higher privileges, and some commands even require root access to run. Unfortunately, you can’t directly execute a command as a separate user, but there are a few things you can do (depending on what’s possible per situation).
Running a Pod as root
To run a Pod as root, you can add the runAsUser option to the securityContext field and set it to the user ID of the root user, which is 0.
https://docs.google.com/document/d/1Pd87rIwF\_bRGFXITdA5hIWpDZmuN1oRu9imcwn1Pbz4/edit
apiVersion: v1
kind: Pod
metadata:
name: web-pod
spec:
securityContext:
runAsUser: 0
containers:
- name: nginx
image: nginx:latest
Applying this will cause the containers within the Pod file to run as root, allowing you to now execute the command as the root user.
Note that you can’t directly edit the security context of a running Pod, so you will either have to edit the deployment if you have one, or edit the Pod file locally and delete then redeploy the changes.
Also note that this option might not work if your security policy in the cluster prevents running Pods as root.
Written by
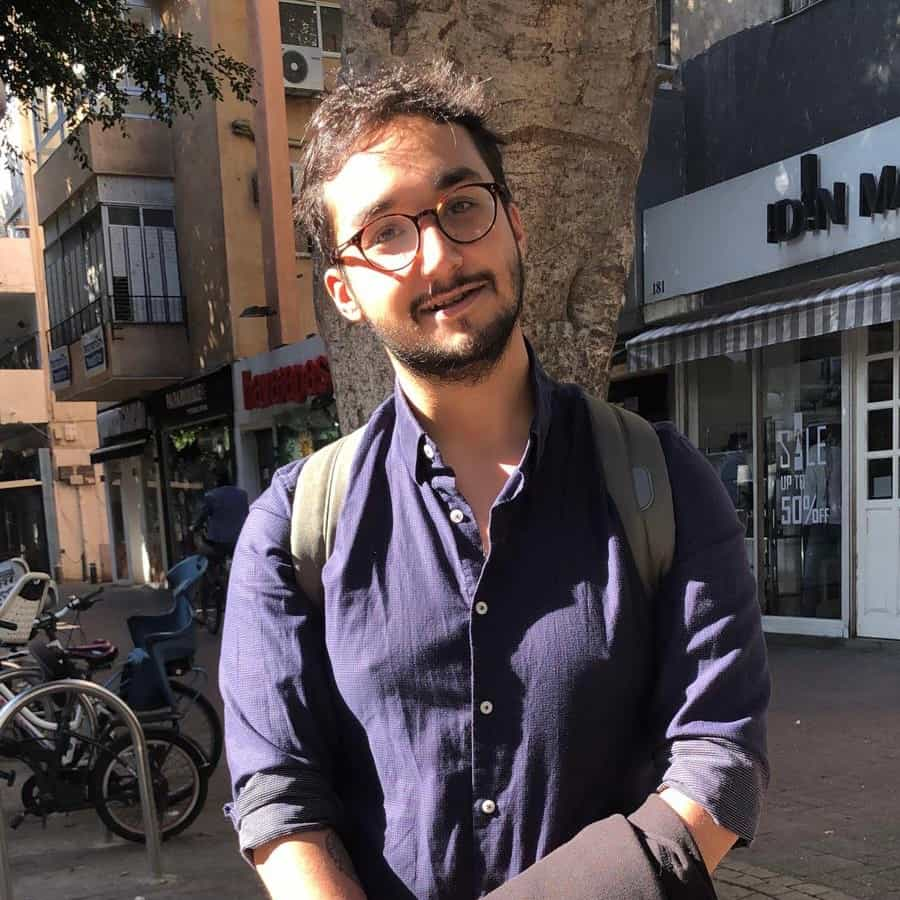
Gabriel Manricks
Chief Architect, ClearX
Filed Under
Related Articles
Copy Files From Pod in Kubernetes
Learn how to copy files and directories from within a Kubernetes Pod into the local filesystem using the kubectl command.
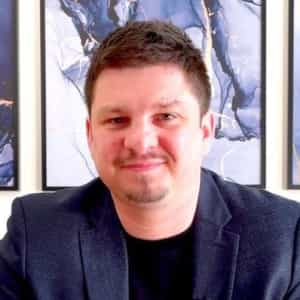
Scale Deployments in Kubernetes
Learn how to manually and automatically scale a Deployment based on CPU usage in Kubernetes using the kubectl-scale and kubectl-autoscale commands.
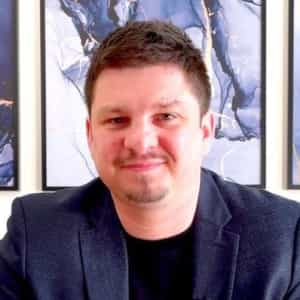
Get Kubernetes Logs With kubectl
Learn how to get the logs of pods, containers, deployments, and services in Kubernetes using the kubectl command. Troubleshoot a cluster stuck in CrashloopBackoff, ImagePullBackoff, or Pending error states.
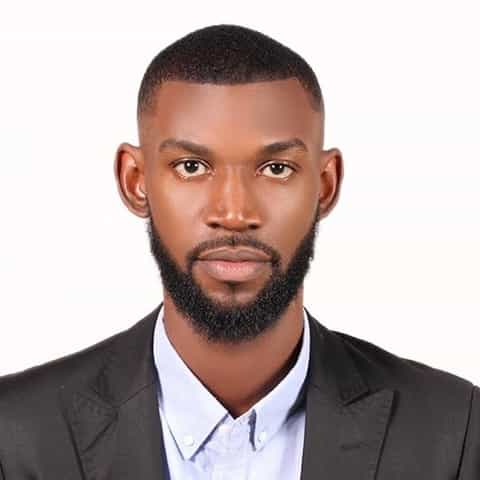
Forward Ports In Kubernetes
Learn how to forward the ports of Kubernetes resources such as Pods and Services using the kubectl port-forward command.
Tail Logs In Kubernetes
Learn how to tail and monitor Kubernetes logs efficiently to debug, trace, and troubleshoot errors more easily using the kubectl command.
Get Context In Kubernetes
Learn how to get information about one or more contexts in Kubernetes using the kubectl command.
Delete Kubernetes Namespaces With kubectl
Learn how to delete one or more namespaces and their related resources in a Kubernetes cluster using the kubectl command.
Get Kubernetes Secrets With kubectl
Learn how to list, describe, customize, sort and filter secrets in a Kubernetes cluster by name, type, namespace, label and more using the kubectl command.
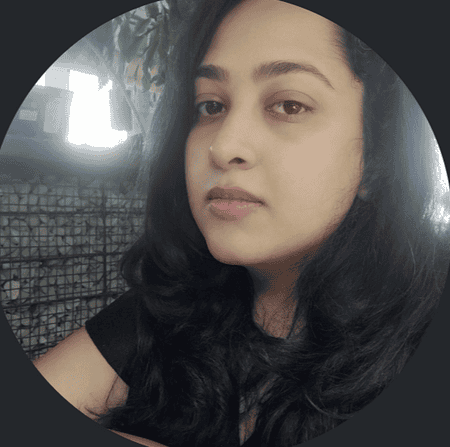
List Kubernetes Namespaces With kubectl
Learn how to list, describe, customize, sort and filter namespaces in a Kubernetes cluster by name, label, and more using the kubectl command.
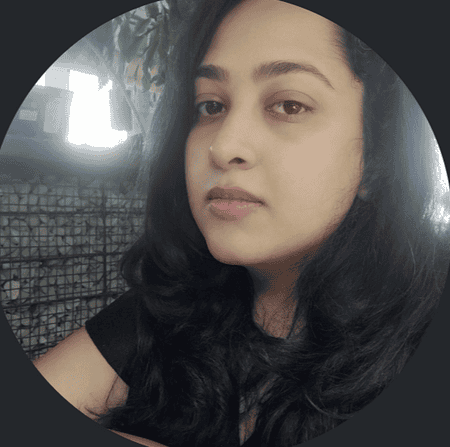
How To List Events With kubectl
Learn how to list and filter events in Kubernetes cluster by namespace, pod name and more using the kubectl command.
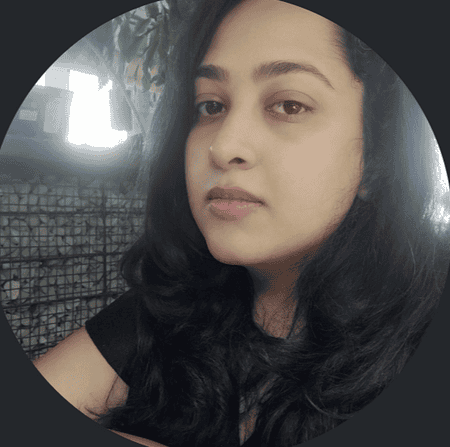
Kubernetes vs Docker: The Backbone of Modern Backend Technologies
Lean the fundamentals of the Kubernetes and Docker technologies and how they interplay with each other.
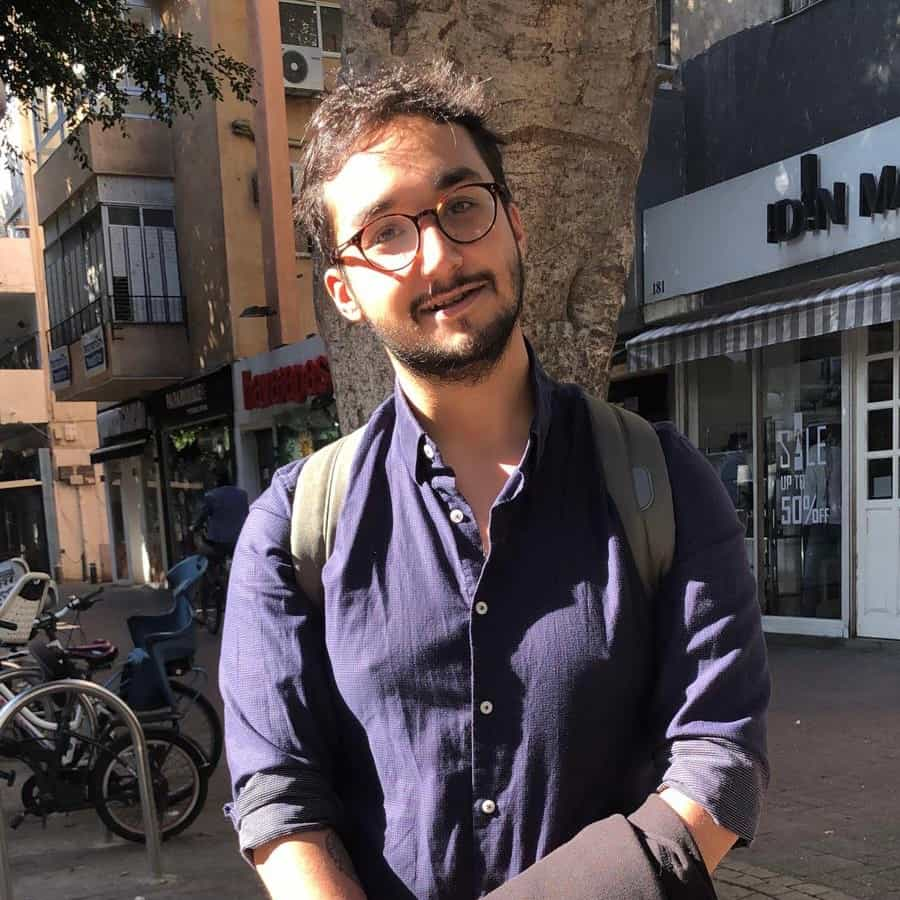
Set Context With kubectl
Learn how to create, modify, switch, and delete a context in Kubernetes using the kubectl config command.
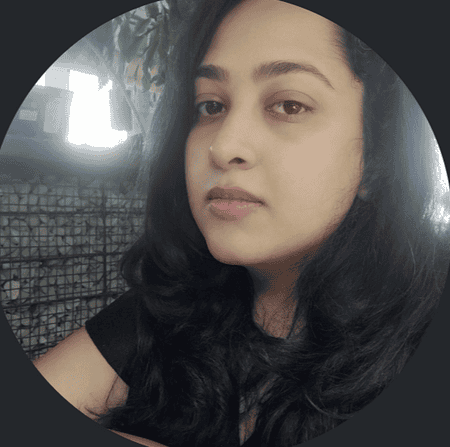