The short answer
To upload a file with curl using the HTTP POST method, you can use the -F flag (short for --form) with the following syntax:
$ curl -F "<form_field>=@<local_file_path>"<upload_url>
Where:
- form_field is the name of the form field that will receive the file.
- local_file_path is the path to the local file you want to upload.
- upload_url is the URL where you want to send the file.
For example:
$ curl -F "[email protected]" https://example.com/upload
This command sends an HTTP POST request to https://example.com/upload with a form field named file containing the contents of the file mydocument.pdf, while setting the Content-Type header to multipart/form-data.
Note that this method is typically used for uploading files via HTTP or HTTPS, and not for FTP or SFTP transfers, which are covered below.
You can learn more about cURL with our other articles on how to send HTTP POST requests with cURL and how to set HTTP headers with cURL.
Easily retrieve this command using Warp’s AI Command Suggestions
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Suggestions feature:
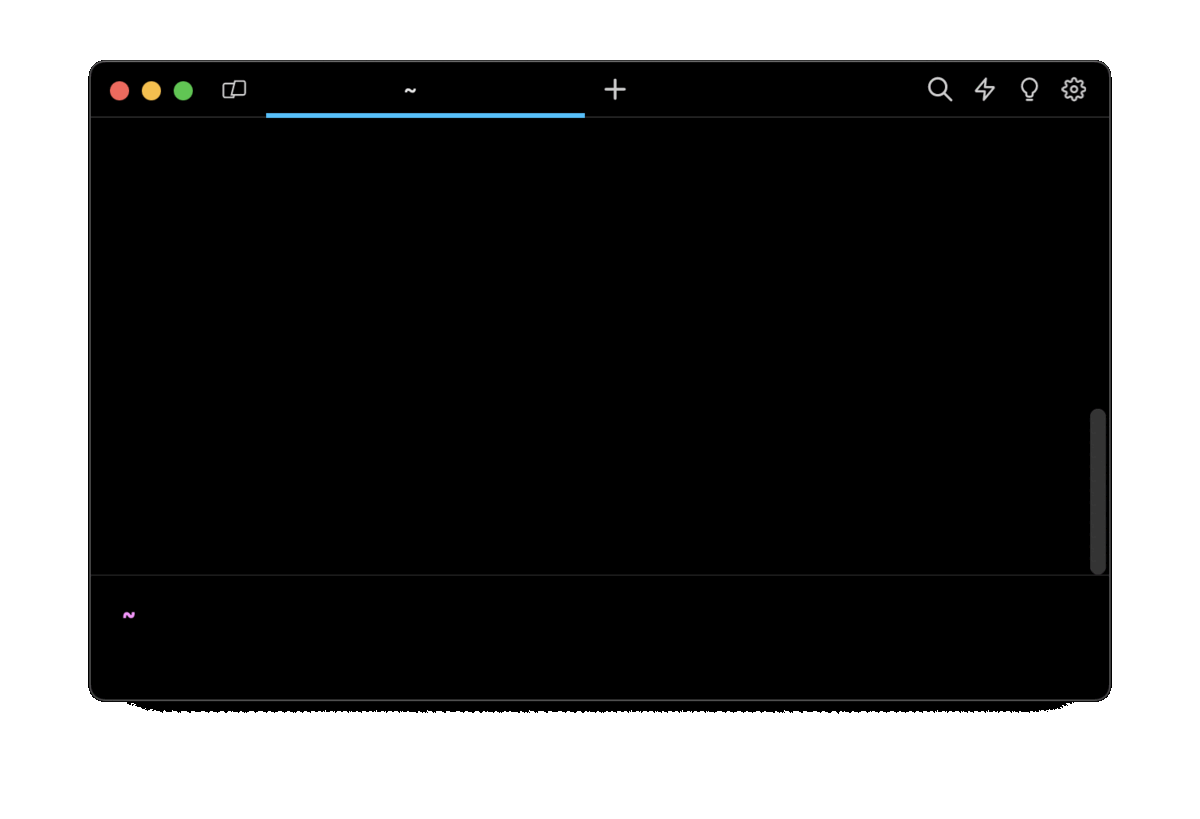
Entering curl upload file in the AI Command Suggestions will prompt a curl command that can then quickly be inserted into your shell by doing CMD+ENTER.
Uploading a file with curl using HTTP PUT
To upload a file using the HTTP PUT method, you can use the curl command with the -T flag (short for --upload-file) as follows:
$ curl -T <local_file_path> <upload_url>
Where:
- local_file_path is the path to the local file you want to upload.
- upload_url is the URL where you want to send the file using the PUT method.
For example:
$ curl -T ./mydocument.txt https://example.com/upload
This command sends an HTTP PUT request to https://example.com/upload with the specified file as the message body of the request.
Uploading a file to an FTP server
To upload a file to an FTP server with curl, you can use the following syntax:
$ curl -T <local_file_path> -u <username>:<password>ftp://<ftp_server_url>/<remote_directory>/
Where:
- local_file_path is the path to the local file you want to upload.
- username is your FTP username.
- password is your FTP password.
- ftp_server_url is the URL of the FTP server you want to connect to.
- remote_directory is the directory on the FTP server where you want to upload the file.
For example:
$ curl -T ./mydocument.txt -u myuser:mypassword ftp://ftp.example.com/uploads/
Note that you should ensure proper permissions and the existence of the remote directory on the FTP server before proceeding. If security is a concern, consider using secure FTP (SFTP) or other encryption methods, as plain FTP transfers data without encryption.
Uploading a file to an SFTP server
To upload a file to an SFTP server with curl, you can use this command syntax:
$ curl -T <local_file_path> sftp://<username>@<sftp_server_url>/<remote_directory>/
Where:
- local_file_path is the path to the local file you want to upload.
- username is your SFTP username.
- sftp_server_url is the URL of the SFTP server you want to connect to.
- remote_directory is the directory on the SFTP server where you want to upload the file.
For example:
$ curl -T ./mydocument.txt sftp://[email protected]/uploads/
Uploading a file to Artifactory
To upload a file to Artifactory with curl, you can use the following command syntax:
$ curl -u <username>:<password> -T "<local_file_path>" "<artifactory_url>/artifactory/<repository>/<target_path>/<filename>"
Where:
- username is your Artifactory username.
- password is your Artifactory password.
- local_file_path is the path to the local file you want to upload.
- artifactory_url is the URL of your Artifactory instance.
- repository is the name of the repository where you want to store the file.
- target_path is the optional directory path within the repository where you want to place the file.
- filename is the name you want to give to the uploaded file.
For example:
$ curl -u myuser:mypassword -T myartifact.jar "https://artifactory.example.com/artifactory/myrepo/myfolder/myartifact.jar"
Uploading a file to an S3 bucket using a presigned URL
To upload a file to an Amazon S3 bucket with curl using a presigned URL, you can use the following command syntax:
$ curl --upload-file <local_file_path> <presigned_url>
Where:
- local_file_path is the path to the local file you want to upload.
- presigned_url is the presigned URL generated for your S3 bucket and object, which includes authentication and expiration information.
For example:
$ curl --upload-file ./mydocument.pdf "https://s3.amazonaws.com/mybucket/myobject?AWSAccessKeyId=YOUR_ACCESS_KEY&Expires=EXPIRATION_TIMESTAMP&Signature=SIGNATURE"
This command sends a PUT request to the presigned URL with the specified file as the request body.
Uploading a file on Windows
To upload a file on a Windows operating system with curl, you can use a command similar to the ones provided earlier, with adjustments for Windows file paths.
Here's the general command structure:
$ curl -F "<form_field>=@C:\path\to\local\file" <upload_url>
Where:
- form_field is the name of the form field that will receive the file.
- C:\path\to\local\file is the Windows file path to the local file you want to upload.
- upload_url is the URL where you want to send the file.
For example:
$ curl -F "file=@C:\Users\Username\Documents\mydocument.pdf" https://example.com/upload
Written by
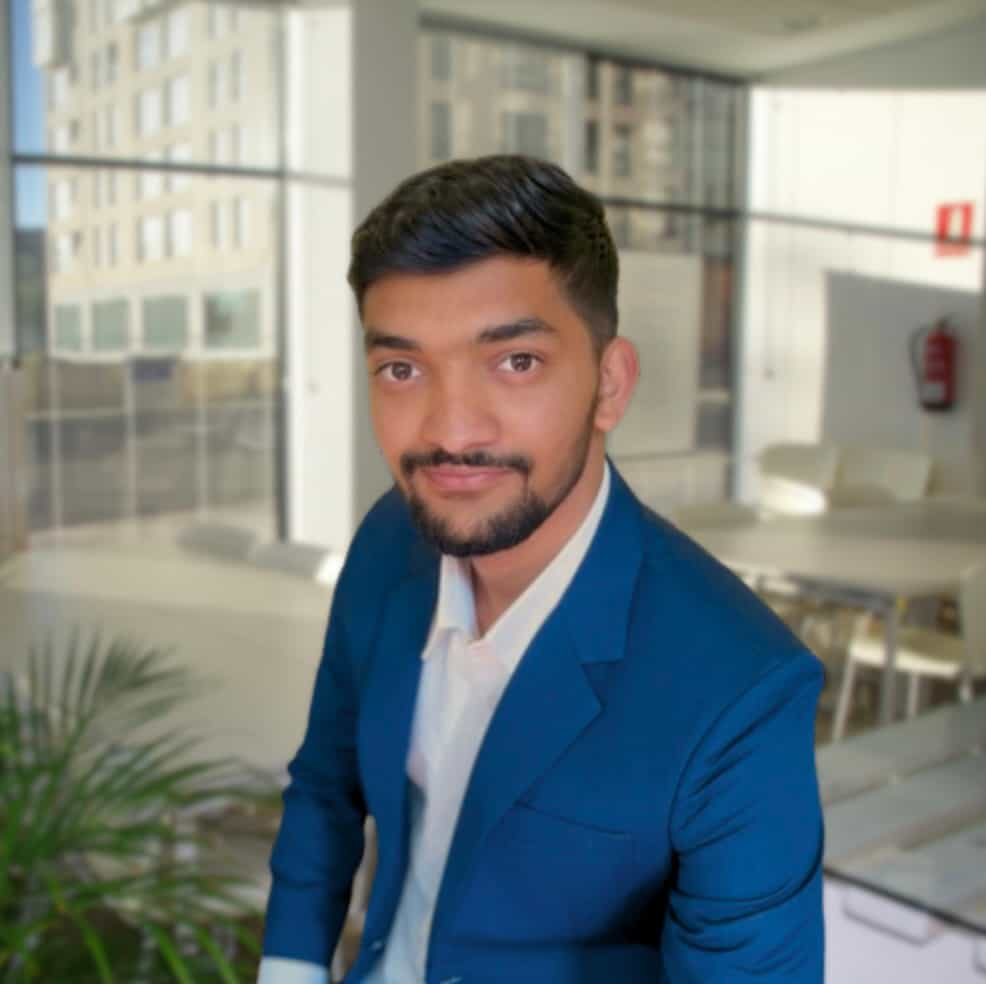
Utsav Poudel
Filed Under
Related Articles
List Open Ports in Linux
Learn how to output the list of open TCP and UDP ports in Linux, as well as their IP addresses and ports using the netstat command.
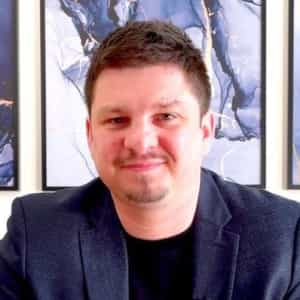
Count Files in Linux
Learn how to count files and folders contained in directories and subdirectories in Linux using the ls, find, and wc commands.
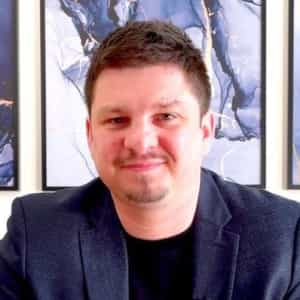
How to Check the Size of Folders in Linux
Learn how to output the size of directories and subdirectories in a human-readable format in Linux and macOS using the du command.
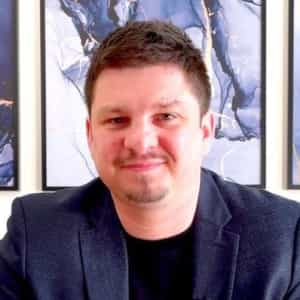
Linux Chmod Command
Understand how to use chmod to change the permissions of files and directories. See examples with various chmod options.
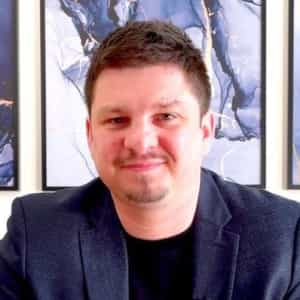
POST JSON Data With Curl
How to send valid HTTP POST requests with JSON data payloads using the curl command and how to avoid common syntax pitfalls. Also, how to solve the HTTP 405 error code.
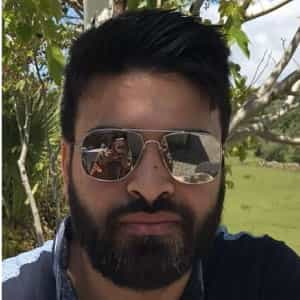
Format Command Output In Linux
Learn how to filter and format the content of files and the output of commands in Linux using the awk command.
Create Groups In Linux
Learn how to manually and automatically create and list groups in Linux.
Switch Users In Linux
Learn how to switch between users, log in as another user, and execute commands as another user in Linux.
Remover Users in Linux
Learn how to remove local and remote user accounts and associated groups and files in Linux using the userdel and deluser commands.
Delete Files In Linux
Learn how to selectively delete files in Linux based on patterns and properties using the rm command.
Find Files In Linux
Learn how to find and filter files in Linux by owner, size, date, type and content using the find command.
Copy Files In Linux
Learn how to safely and recursively copy one or more files locally and remotely in Linux using the cp and scp command.