The short answer
In Docker Compose, a network refers to a layer allowing the containers of the services defined in the compose.yaml file to communicate with each other.
To create a network, you can set the networks attribute and assign it to multiple services in the Compose file as follows:
services:
db:
image: postgres
networks:
- <network_name>
networks:
<network_name>:
Where:
- The top-level networks attribute creates the network named network_name.
- The networks attribute in specific services refers to using the aforementioned network_name.
For example:
services:
db:
image: postgres
networks:
- db_network
web:
image: webapp
networks:
- db_network
- app_network
frontend:
image: frontend
networks:
- app_network
networks:
app_network:
db_network:
In this example, when you run the docker compose up command:
- Two networks named db_network and app_network will be created.
- The containers of the db service will be accessible within the db_network network.
- The containers of the frontend service will be accessible within the app_network network.
- The containers of the web service will be accessible in both the db_network and app_network networks.
- The service db is isolated from the frontend service as they do not share a common network.
Easily retrieve this syntax using Warp’s AI
If you’re using Warp as your terminal, you can easily retrieve this syntax using the Warp AI feature:
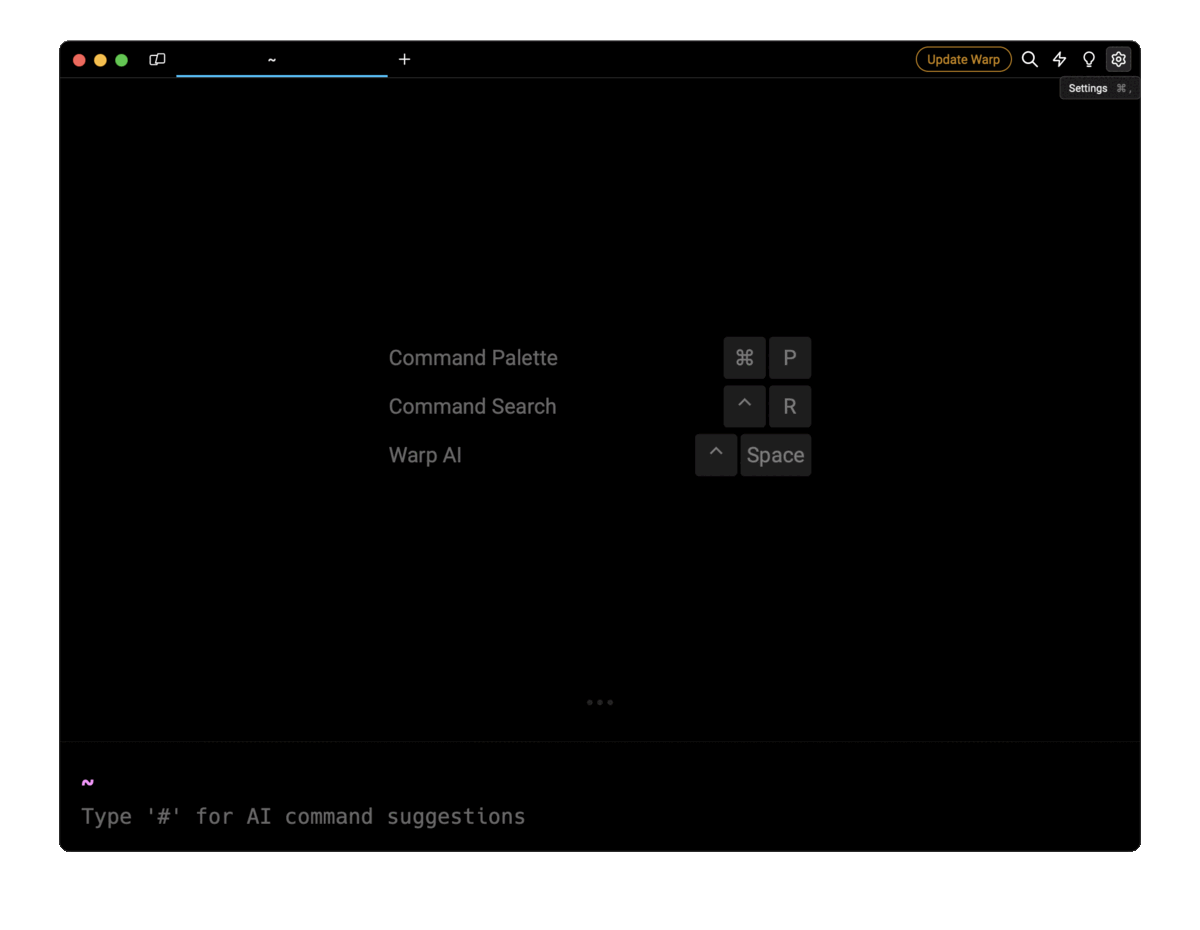
Entering docker compose networks in the AI question input will prompt a human-readable step by step guide including code snippets.
Specifying a driver for networks
In Compose, network drivers control how containers communicate by setting up connections with user-defined networks, external networks, help accessing shared storage resources, and more.
To use a driver for networks, you can specify the driver attribute in the top-level networks section as follows:
services:
app:
image: app
networks:
- my_network
networks:
my_network:
driver: <driver_name>
Where:
- driver_name refers to the specific driver you want to use, like host, overlay, bridge, etc.
For example:
services:
app:
image: app
networks:
- my_network
networks:
my_network:
driver: overlay
In this example, the network named my_network is configured to use a driver named overlay, which allows the containers of the app service to communicate with other containers located on different hosts.
Note that, Compose will return an error if the specified driver is unavailable on the target platform. You can refer to the official documentation to learn more about Docker’s network drivers.
Overriding the default network driver
By default, when no specific network is specified in the compose.yaml file, Compose creates a default network using the default driver bridge, which allows communication between the containers on the same host.
To override the default driver, you can specify the default keyword in the top-level networks attribute as follows:
# project directory name “myapp”
services:
web:
image: web
db:
image: postgres
networks:
default:
driver: custom-driver
In this example, when you run the docker compose up command:
- A default network named myapp_default will be created, which is based on your project directory’s name myapp.
- The myapp_default network will be configured to use the custom driver named custom-driver and is accessible to the containers of both services web and db.
Specifying a custom IPAM configuration
In Compose, the IP Address Management (IPAM) configures the network, controlling how IP addresses are assigned to the containers within the network to prevent conflicts or connectivity issues.
To specify a custom IPAM configuration, you can use the ipam attribute in your compose.yaml file as follows:
services:
webapp:
image: webapp
networks:
- my_network
networks:
my_network:
ipam:
driver: <driver>
config:
- subnet: <subnet>
ip_range: <ip_range>
gateway: <gateway>
aux_addresses:
<hostname>: <value>
options:
<key>: <value>
Where:
- The driver attribute (optional) refers to a custom IPAM driver.
- Under the config attribute (optional), you can specify one or more configurations:
- subnet refers to a Subnet in CIDR (Classless Inter-Domain Routing) format.ip_range refers to the range of IPs used to allocate container IPs. gateway refers to the IPv4 or IPv6 gateway for the specified Subnets. aux_addresses refers to auxiliary IPv4 or IPv6 addresses, where the value represents the auxiliary IP address assigned to the corresponding host identified by hostname.
- The options are driver-specific configurations provided as a key-value mapping.
For example:
services:
webapp:
image: webapp
networks:
- my_network
networks:
my_network:
ipam:
driver: custom-driver
config:
- subnet: 172.28.0.0/16
ip_range: 172.28.5.0/24
gateway: 172.28.5.254
aux_addresses:
host1: 172.28.1.5
host2: 172.28.1.6
options:
level: 0
In this example, a custom IPAM configuration for the network named my_network is specified:
- The IPAM driver is set to custom-driver.
- The configuration is set as follows:
- The subnet is set to 172.28.0.0/16, indicating that the network can accommodate IP addresses ranging from 172.28.0.0 to 172.28.255.255.
- The ip_range is set to 172.28.5.0/24 specifying the range of IPs for the containers.
- The gateway is set to 172.28.5.254, indicating that the containers can use this IP address as a default gateway for the specified Subnets.
- The aux_addresses indicates that the host1 and host2 are assigned the auxiliary addresses 172.28.1.5 and 172.28.1.6 respectively.
- The options are driver-specific, and the key label with value 0 will be used for the specified driver custom-driver.
Setting a static IP address
To set a static IP address, you can use the ipv4_address or ipv6_address attributes as follows:
services:
webapp:
image:webapp
networks:
my-network:
ipv4_address: <ipv4_address>
ipv6_address: <ipv6_address>
networks:
my-network:
Where:
- ipv4_address or ipv6_address is the desired IPv4 or IPv6 address.
For example:
services:
webapp:
image: webapp
networks:
my-network:
ipv4_address: 172.16.238.10
ipv6_address: 2001:3984:3989::10
networks:
my-network:
ipam:
config:
- subnet: "172.16.238.0/24"
- subnet: "2001:3984:3989::/64"
In this example:
- The webapp service is assigned static IPv4 address 172.16.238.10 and static IPv6 address 2001:3984:3989::10.
- The ipam attribute with the subnet configuration in the top-level networks section specifies the address ranges.
Connecting to external networks
To connect the containers of your current Compose file with ones outside that file, you can use the external attribute under the top-level networks attribute, and connect to networks defined in another Compose file as follows:
services:
app:
image: app
networks:
- my_network
networks:
my_network:
name: <external_network>
external: true
Where:
- external_network is the network’s name outside the scope of the Compose file.
For example:
# Redis
services:
db:
image: redis:latest
networks:
- my_network
networks:
my_network:
driver: bridge
name: redis_network
# Web application
services:
my_app:
image: web-app:latest
networks:
- my-app
networks:
my-app:
name: redis_network
external: true
In this example, we have created a shared network for a Redis cache and a web application:
- The Compose file for the Redis application defines a network named redis_network with a bridge driver, making it available for the containers of the db service.
- The Compose file for the web application uses the external attribute with a value of true to establish a connection with the network created outside the scope of the Compose file, in this case the redis_network.
As another example, you can connect with the Docker’s built-in host or none network as follows:
# host
services:
app:
image: app
networks:
- hostnet:
networks:
hostnet:
external: true
name: host
# none
services:
app:
image: app
networks:
nonet:
networks:
nonet:
external: true
name: none
In these examples:
- The app service is connected to a Docker’s built-in network named host and none, which are outside the scope of the Compose files.
- The host network specifies that containers share the network directly with the host and is helpful for high-performance applications where you need to handle large volumes of data, such as real-time analytics.
- The none network turns off the networking and specifies that containers run entirely in isolation from the host and other containers.
- The alias hostnet and nonet are used explicitly for Docker's built-in host and none networks.
Written by
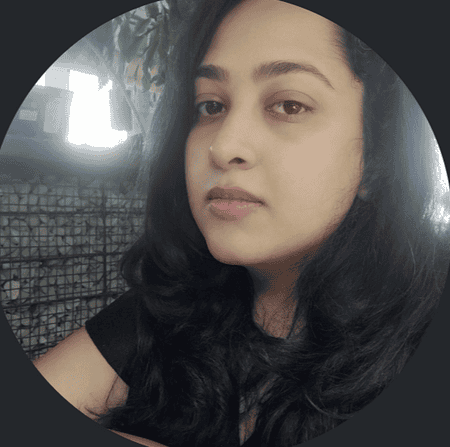
Mansi Manhas
Filed Under
Related Articles
Override the Container Entrypoint With docker run
Learn how to override and customize the entrypoint of a Docker container using the docker run command.
The Dockerfile ARG Instruction
Learn how to define and set build-time variables for Docker images using the ARG instruction and the --build-arg flag.
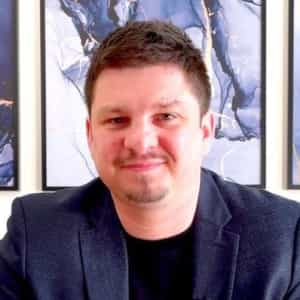
Start a Docker Container
Learn how to start a new Docker container from an image in both the foreground and the background using the docker-run command.
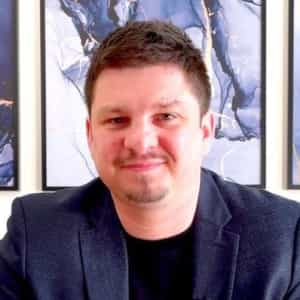
Stop All Docker Containers
How to gracefully shutdown running containers and forcefully kill unresponsive containers with signals in Docker using the docker-stop and docker-kill commands.
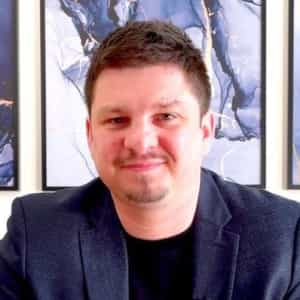
Use An .env File In Docker
Learn how to write and use .env files in Docker to populate the environment of containers on startup.
Run SSH In Docker
Learn how to launch and connect to a containerized SSH server in Docker using password-based authentication and SSH keys.
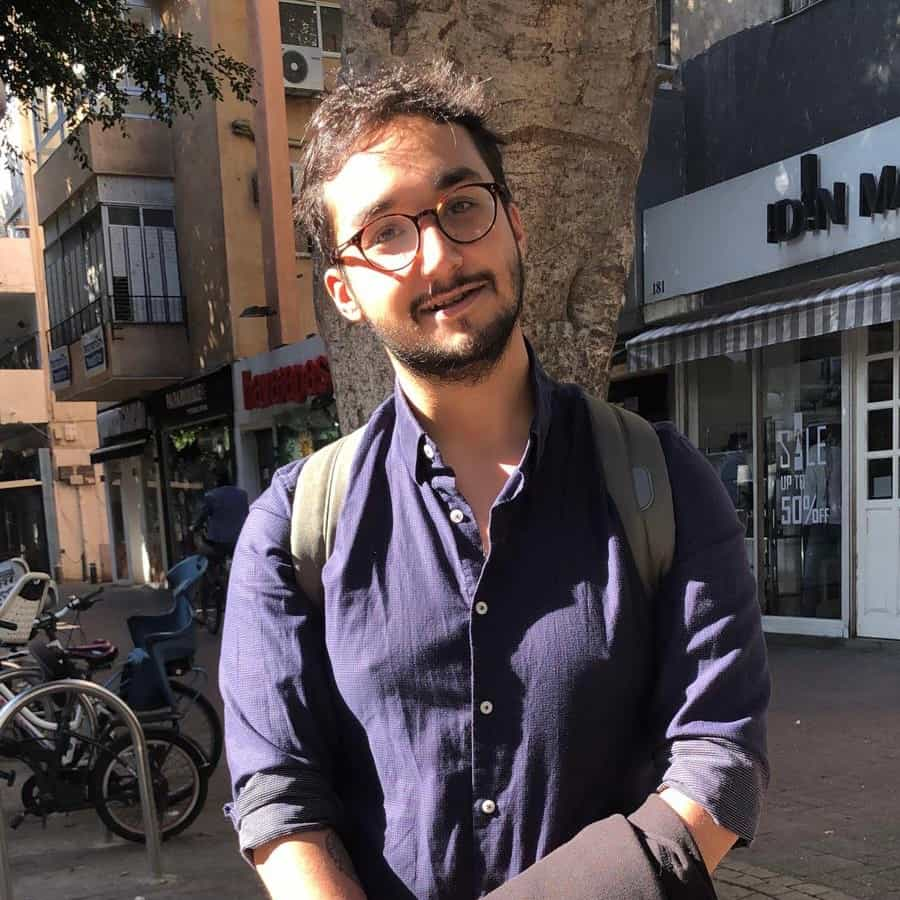
Launch MySQL Using Docker Compose
Learn how to launch a MySQL container in Docker Compose.
Execute in a Docker Container
Learn how to execute one or multiple commands in a Docker container using the docker exec command.
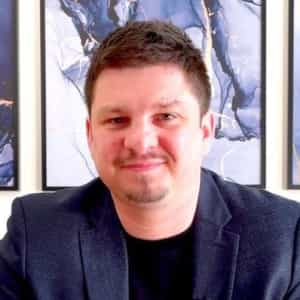
Expose Docker Container Ports
Learn how to publish and expose Docker container ports using the docker run command and Dockerfiles.
Restart Containers In Docker Compose
Learn how to restart and rebuild one or more containers in Docker Compose.
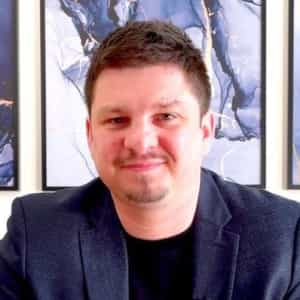
Output Logs in Docker Compose
Learn how to output, monitor, customize and filter the logs of the containers related to one or more services in Docker Compose
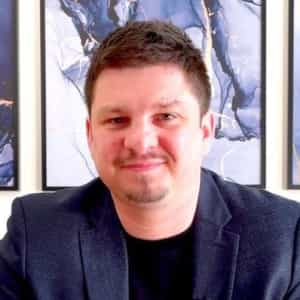
Rename A Docker Image
Learn how to rename Docker images locally and remotely using the docker tag command.