The short answer
To set up a MySQL database container in Docker Compose, you can start by creating a compose.yaml file within your project's directory with the following content:
version: '3'
services:
database:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: <root_password>
MYSQL_DATABASE: <database_name>
MYSQL_USER: <user_name>
MYSQL_PASSWORD: <user_password>
ports:
- 3306:3306
Where:
- root_password is the required password for the MySQL root account.
- database_name is the name for the default database that the new container creates when it starts.
- user_name and user_password are optional environment variables that you can use altogether to create a new user with superuser privileges for the database specified in database_name .
Then run the following docker compose command to start the MySQL container:
$ docker compose -f compose.yaml up -d
Where:
- The -f option specifies the Compose file’s path.
- The -d option launches the services in detached mode (i.e. in the background).
If you want to learn how to do the same thing with PostgreSQL, you can read our other article on how to launch PostgreSQL using Docker Compose.
Easily retrieve this syntax using Warp AI
If you’re using Warp as your terminal, you can easily retrieve this syntax using the Warp AI feature:
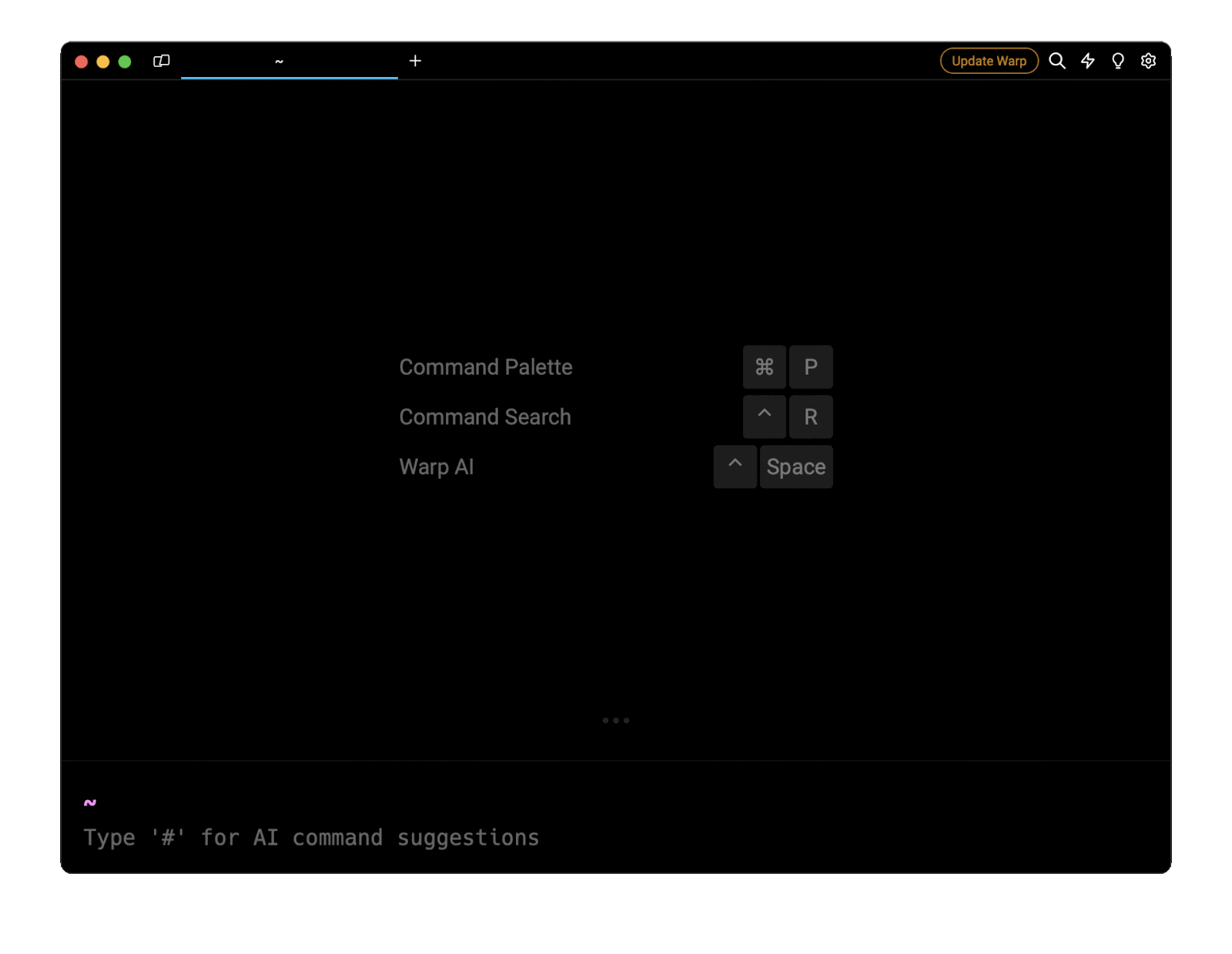
For example, entering docker compose mysql in the AI question input will prompt a human-readable step by step guide including code snippets.
Persisting the container's data with named volumes
By default, all the data generated in the MySQL container will be lost upon termination.
To prevent this data loss, you can use the top-level volumes property in your compose.yaml file to create a named volume, which is a persistent storage mechanism provided by Docker:
version: '3'
services:
database:
image: mysql:latest
environment:
MYSQL_ROOT_PASSWORD: <root_password>
MYSQL_DATABASE: <database_name>
MYSQL_USER: <user_name>
MYSQL_PASSWORD: <user_password>
ports:
- 3306:3306
volumes:
- <volume_name>:<volume_location>
volumes:
<volume_name>:
Where:
- <volume_name> is the name of the volume the container's data will be persisted on.
- <volume_location> is the path where the volume will be mounted within the container. By default, /var/lib/mysql .
For example:
version: '3'
services:
database:
image: mysql:latest
environment:
MYSQL_ROOT_PASSWORD: password102
MYSQL_DATABASE: my-database
MYSQL_USER: emminex
MYSQL_PASSWORD: password104
ports:
- 3306:3306
volumes:
- mysql_data:/var/lib/mysql
volumes:
mysql_data:
You can learn more about persisting data in Docker Compose by reading the official documentation page on named volumes and our other article on how to create a bind mount in Docker Compose.
Initializing the database using scripts
When running a new database instance, it is sometimes necessary to initialize it by automating the creation of tables, populating it with a default dataset, or customizing its configuration before other applications can connect to it.
To do so, you can add all your initialization scripts (i.e., .sql , .sh , or .sql.gz ) in a directory on your local machine and bind it to the MySQL container's /docker-entrypoint-initdb.d directory through the volumes property as follows:
version: '3'
services:
database:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: <root_password>
MYSQL_DATABASE: <database_name>
MYSQL_USER: <user_name>
MYSQL_PASSWORD: <user_password>
ports:
- 3306:3306
volumes:
- <scripts>:/docker-entrypoint-initdb.d
Where:
- scripts is the path to the directory on your local machine containing the initialization scripts.
Upon startup, all the scripts located in the docker-entrypoint-initdb.d directory will automatically be executed by the container.
Automatically restarting the database on failure
To automatically restart your MySQL container in case of failure or unexpected behavior, you can set the restart property to always , which will ensure that your container keep running forever until it is manually stopped.
For example:
version: '3'
services:
database:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: ${MYSQL_ROOT_PASSWORD}
MYSQL_DATABASE: ${MYSQL_DATABASE}
MYSQL_USER: ${MYSQL_USER}
MYSQL_PASSWORD: ${MYSQL_PASSWORD}
ports:
- 3306:3306
restart: always
You can learn more about restart policies in Compose by reading our other article on how to restart containers in Docker Compose https://www.warp.dev/terminus/docker-compose-restart.
Expressing a dependency between MySQL and other services
To ensure that the database container is up and running before the various services defined in the compose.yaml file are launched, you can create a dependency between them using the depends_on property.
In this example, Compose will first launch the database service, then wait for it to be up and running before launching the website service:
version: '3'
services:
website:
image: nginx
depends_on:
- db
database:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: password102
MYSQL_DATABASE: my-database
MYSQL_USER: emminex
MYSQL_PASSWORD: password104
ports:
- 3306:3306
Ensuring a healthy database connection
Although the depends_on property tells Compose to start the MySQL container first, it doesn’t guarantee that the database is fully ready to handle requests.
To solve this issue, you can use the healthcheck property to define a condition that will determine whether it is safe for other services to attempt to connect to the database.
In this example, Compose will first launch the database service, then execute the command defined in the healthcheck.test property every 10 seconds to verify the service's readiness, and finally launch the website service once the command is deemed successful:
version: '3'
services:
website:
image: nginx
depends_on:
database:
condition: service_healthy
database:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: password102
MYSQL_DATABASE: my-database
MYSQL_USER: emminex
MYSQL_PASSWORD: password104
ports:
- 3306:3306
healthcheck:
test: ["CMD", "mysqladmin", "ping", "-h", "localhost"]
interval: 10s
timeout: 5s
retries: 5
You can learn more startup conditions in Compose by reading our other article on how to perform a health check in Docker Compose.
Keeping the database credentials safe with .env files
To prevent the accidental leak of sensitive information such as database credentials, it is considered good practice to keep them outside of your Compose configuration by placing them in an environment file that is not pushed to your repository.
To do so, you can first create a new .env file within the same directory as your compose.yaml file containing your MySQL credentials:
MYSQL_ROOT_PASSWORD=password102
MYSQL_DATABASE=my-database
MYSQL_USER=emminex
MYSQL_PASSWORD=password104
Then replace the values of the environment variables in your compose.yaml file using the variable interpolation syntax:
version: '3'
services:
database:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: ${MYSQL_ROOT_PASSWORD}
MYSQL_DATABASE: ${MYSQL_DATABASE}
MYSQL_USER: ${MYSQL_USER}
MYSQL_PASSWORD: ${MYSQL_PASSWORD}
ports:
- 3306:3306
When running the docker compose up command, Compose will automatically replace those variables at runtime.
You can learn more about using environment variables in Compose by reading our other article on how to use an .env file in Docker Compose.
Written by
Emmanuel Oyibo
Filed Under
Related Articles
Override the Container Entrypoint With docker run
Learn how to override and customize the entrypoint of a Docker container using the docker run command.
The Dockerfile ARG Instruction
Learn how to define and set build-time variables for Docker images using the ARG instruction and the --build-arg flag.
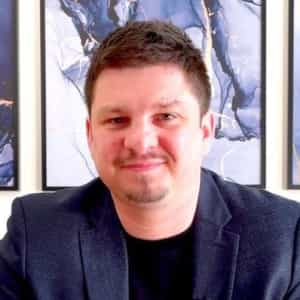
Start a Docker Container
Learn how to start a new Docker container from an image in both the foreground and the background using the docker-run command.
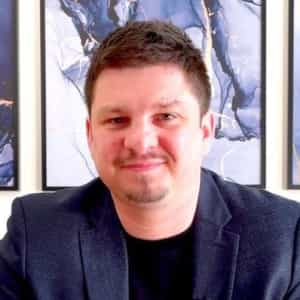
Stop All Docker Containers
How to gracefully shutdown running containers and forcefully kill unresponsive containers with signals in Docker using the docker-stop and docker-kill commands.
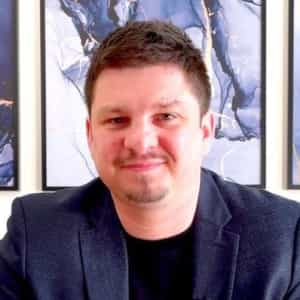
Use An .env File In Docker
Learn how to write and use .env files in Docker to populate the environment of containers on startup.
Run SSH In Docker
Learn how to launch and connect to a containerized SSH server in Docker using password-based authentication and SSH keys.
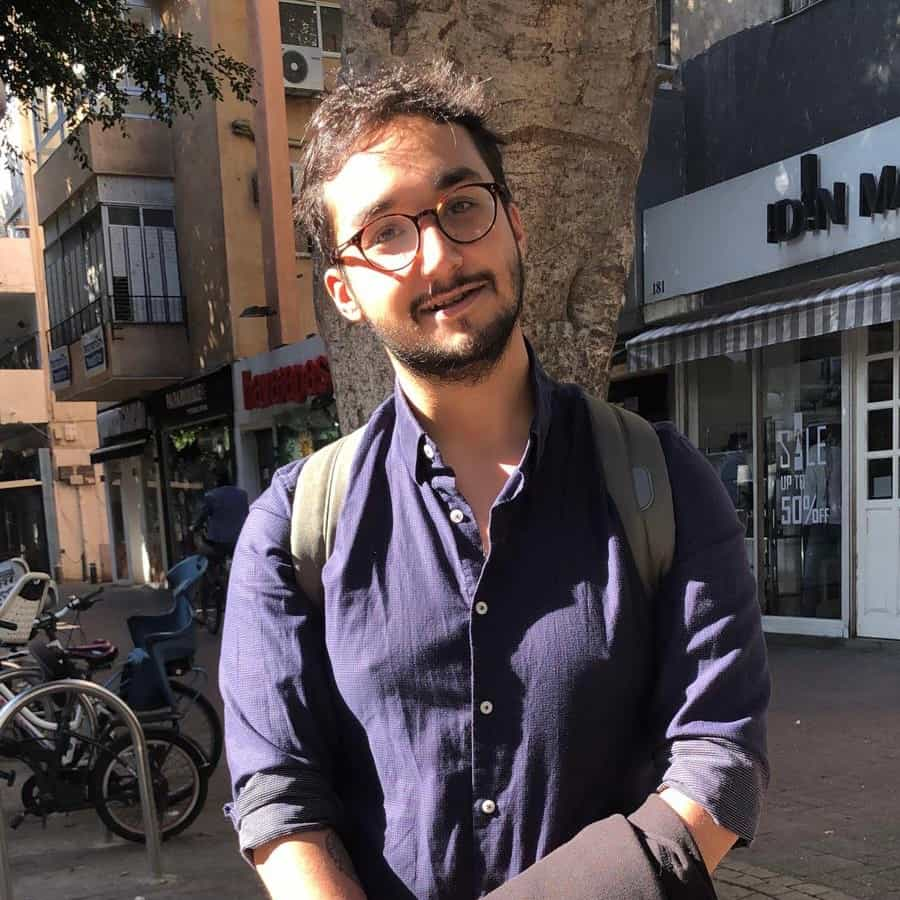
Execute in a Docker Container
Learn how to execute one or multiple commands in a Docker container using the docker exec command.
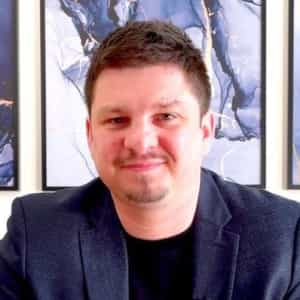
Expose Docker Container Ports
Learn how to publish and expose Docker container ports using the docker run command and Dockerfiles.
Restart Containers In Docker Compose
Learn how to restart and rebuild one or more containers in Docker Compose.
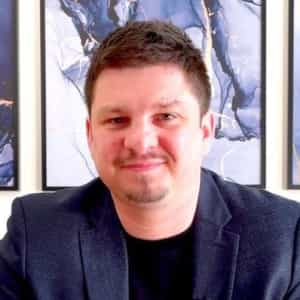
Output Logs in Docker Compose
Learn how to output, monitor, customize and filter the logs of the containers related to one or more services in Docker Compose
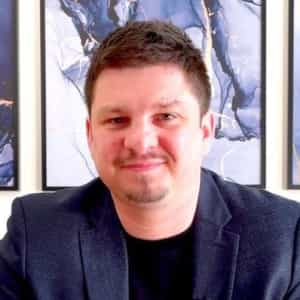
Rename A Docker Image
Learn how to rename Docker images locally and remotely using the docker tag command.
Understand healthcheck in Docker Compose
Learn how to check if a service is healthy in Docker Compose using the healthcheck property.
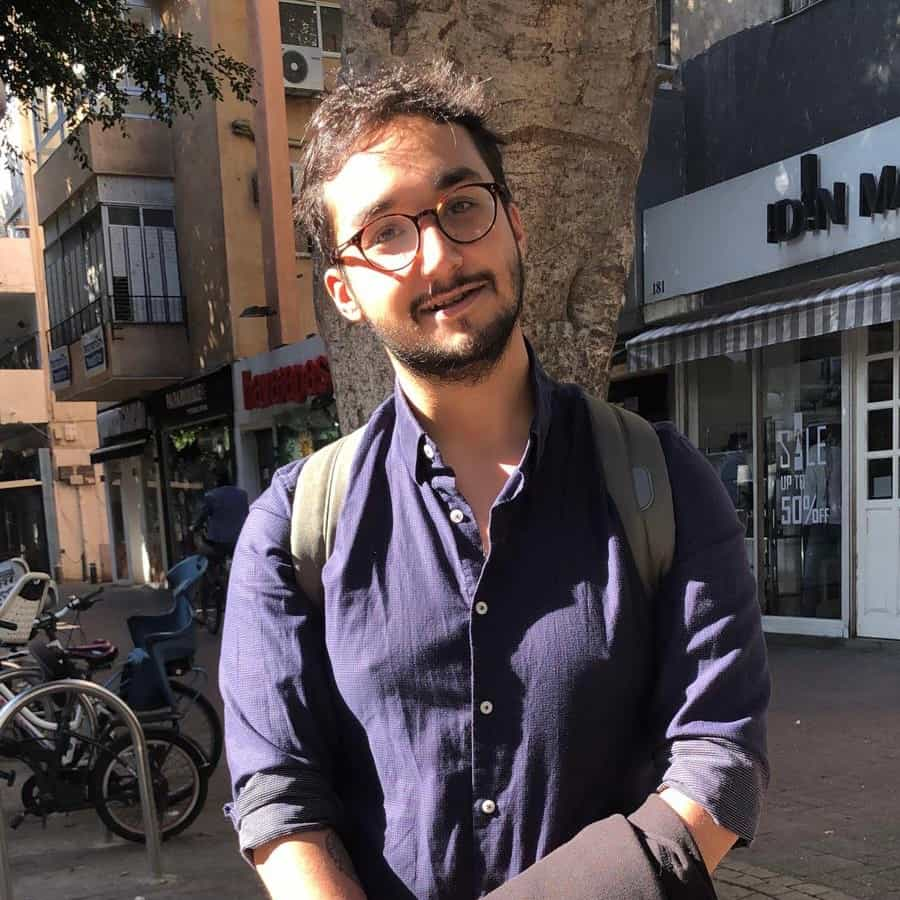