There are a few different ways your bash program can accept user input
- 1. Using Command Line Arguments
- 2. Prompt for input during script execution
Accepting User Input with Command Line Arguments
When you run a command on the command line, you can supply arguments to them that act as inputs for that command.
For instance, when you run ls -l /Documents, the -l
and /Documents
are both command line arguments to the command ls
.
You can do the same thing while writing your own bash scripts. For example, by passing "Amit" and "dog" as positional parameter arguments -
bash print-name-animal.sh amit dog

When you run this command, bash will automatically provide the following positional parameter variables to our script, each containing the contents of the command line.
- $0 - The variable $0 is set to the first word of the command line statement - the name of your bash script. In this case, it will be print-name-animal.sh
- $1 - First parameter after the command name, in this case it will be set to amit
- $2 - Second parameter after the command name, in this case it will be set to dog
If there was a third parameter, its value would be placed in the variable $3, and so on.
These shell variables are automatically set by the shell when we run our script so all we need to do is refer to them in our script, like:
print-name-pet.sh
#!/bin/bash
# a simple bash script that accepts user's name and favorite pet animal as command line arguments and prints them
echo "Your name is $1"
echo "Your favorite pet animal is $2"
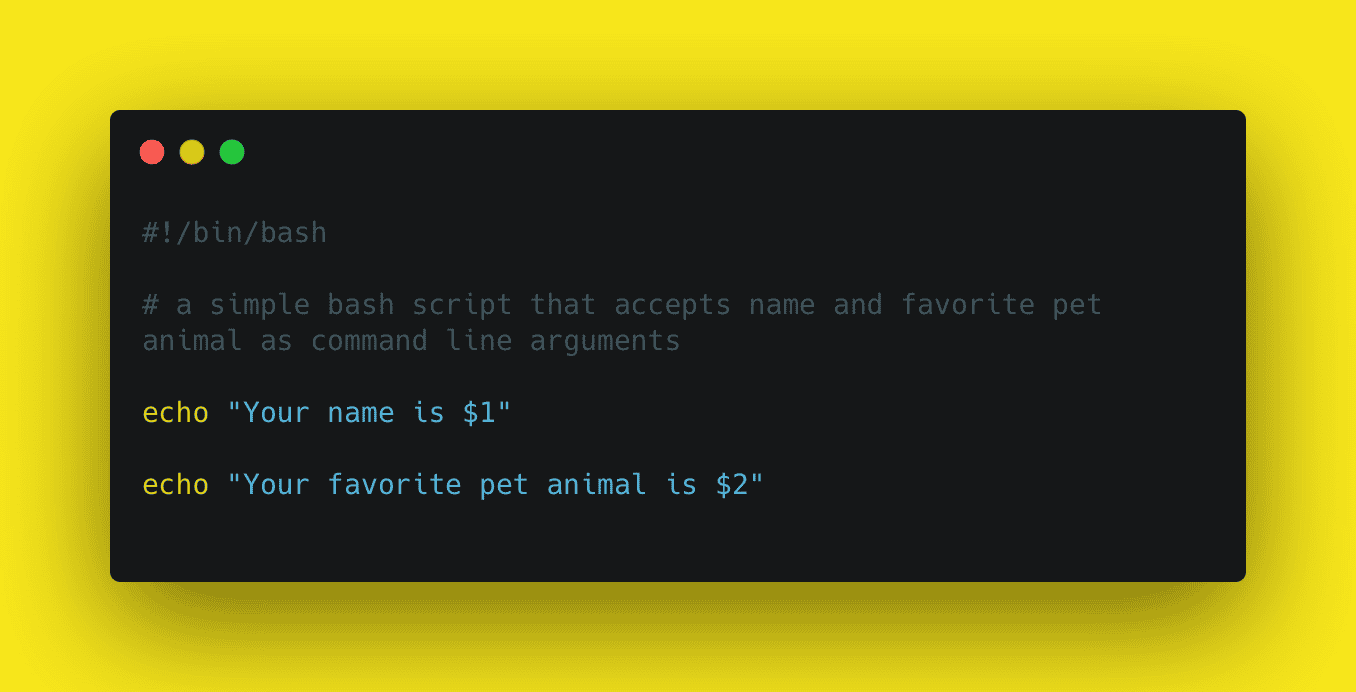
But, what if you had more than 9 parameters?
Parameters greater than 9 can be accessed using curly braces around the number; for instance, ${10} would be the tenth parameter, and ${123} would be the 123rd.
Did you know?
You can also check the number of positional parameters (excluding $0) by using the variable $#.
You can also access all the items on the command line starting at the first parameter ($1), with the special variable $@.
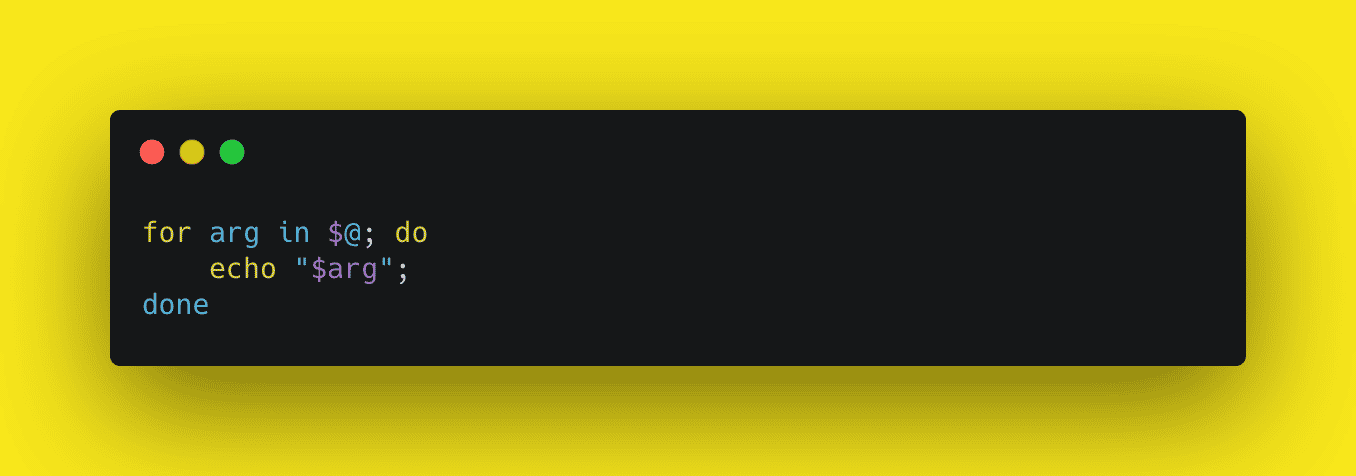
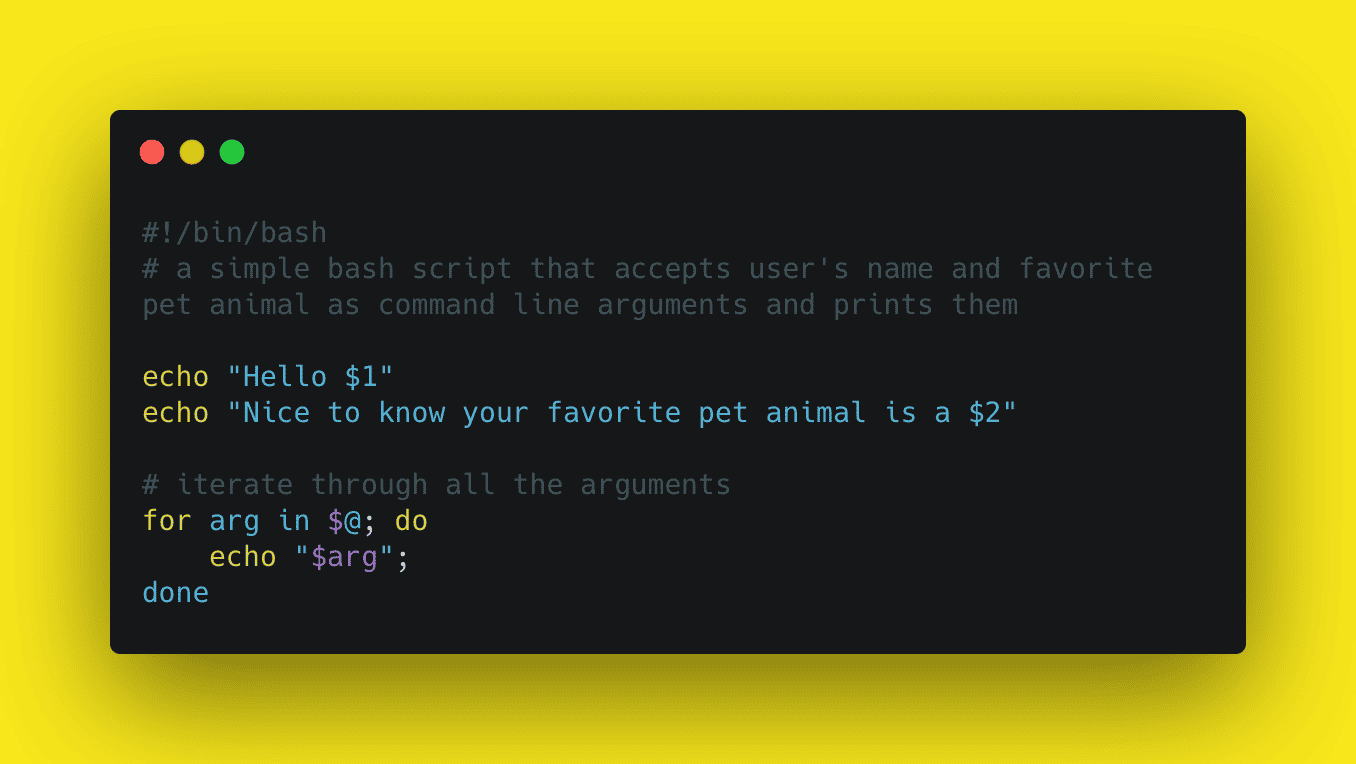
Best Practices
Positional parameters are delimited by a space. The shell interprets the things after the spaces as individual parameters. If the parameter itself contains a space, enclose it in quotation marks, like "Snow Leopard".
Prompt for input using read
In addition to using command line arguments as input, you can also prompt the user for input during the script execution. You do that using the read command.
The syntax for the read command is
read-p "PROMPT" VARIABLE_NAME
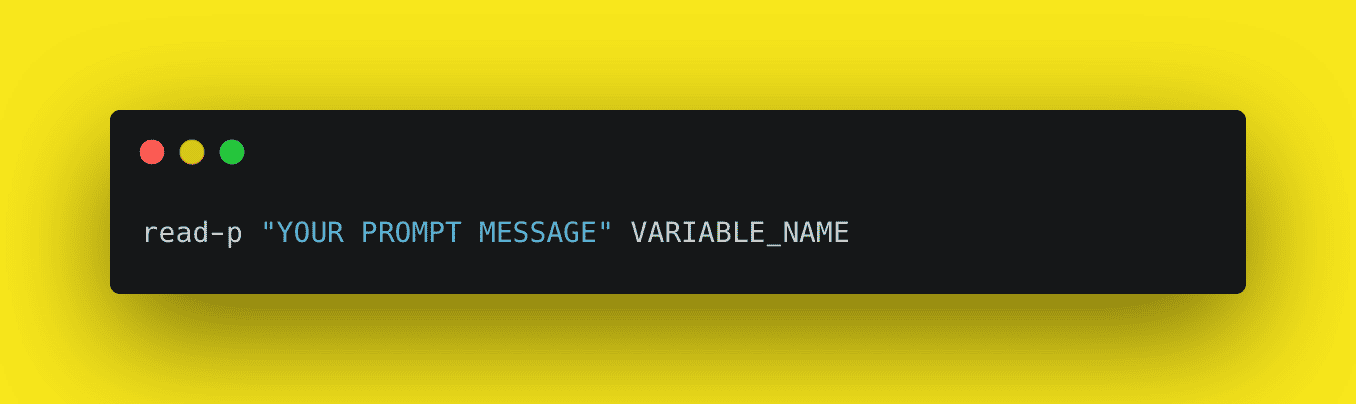
Here’s an example for using it ask the user for their name and favorite pet:
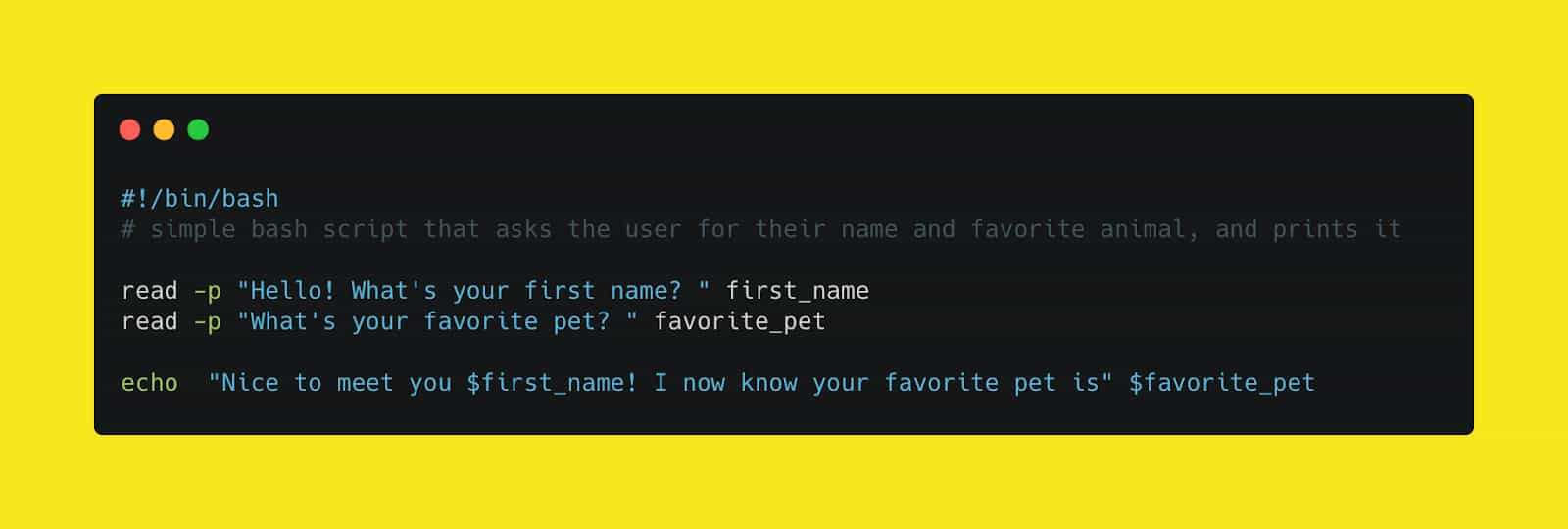
#!/bin/bash
# simple bash script that asks the user for their name and favorite animal, and prints it
read -p "Hello! What's your first name? " first_name
read -p "What's your favorite pet? " favorite_pet
echo "Nice to meet you $first_name! I now know your favorite pet is" $favorite_pet
How it works
The read command here captures the input from the user and stores it in our variables - $first_name and $favorite_animal
Read Command Options
The read command provides a few options/flags you can use:
- -p Prompt: You can use this to provide a helpful message for the user before the input prompt. For example, read -p “What’s your height (inches)?” user_height
- -s Silent Mode: You can use this to accept invisible input from the user. Useful for passwords or other secret information.
- -t Timed Input: You can use this to set time in seconds that your script should wait for taking input from the user.
Choosing Command Line Arguments vs Prompting for execution
Accept user input through command line arguments (preferred, stays in history, making it easier for users to run the command again, without waiting for manual typing for input)
Written by
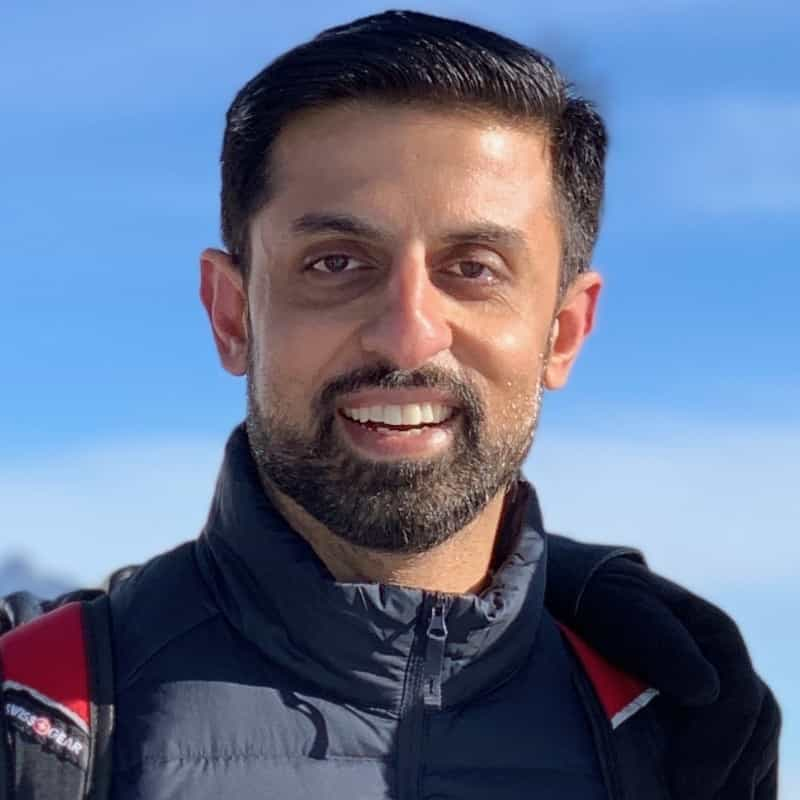
Amit Jotwani
Developer Relations, Warp
Filed Under
Related Articles
Bash If Statement
Learn how to use the if statement in Bash to compare multiple values and expressions.
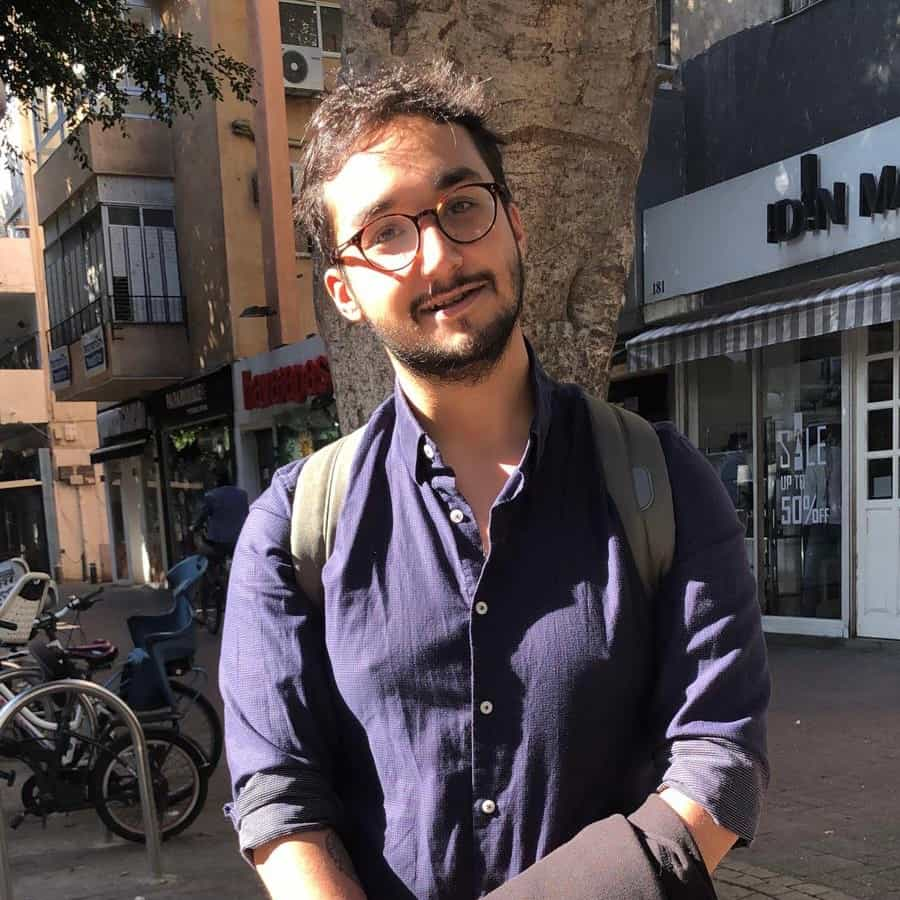
Bash While Loop
Learn how to use and control the while loop in Bash to repeat instructions, and read from the standard input, files, arrays, and more.
POST JSON Data With Curl
How to send valid HTTP POST requests with JSON data payloads using the curl command and how to avoid common syntax pitfalls. Also, how to solve the HTTP 405 error code.
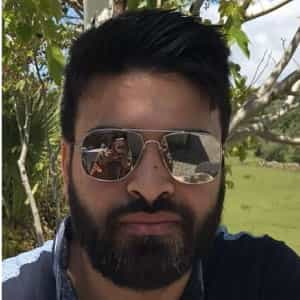
Use Cookies With cURL
Learn how to store and send cookies using files, hard-coded values, environment variables with cURL.
Loop Through Files in Directory in Bash
Learn how to iterate over files in a directory linearly and recursively using Bash and Python.
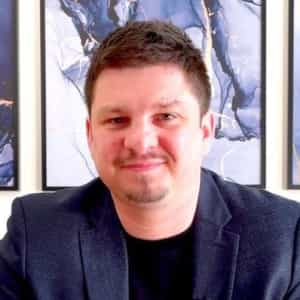
How To Use sudo su
A quick overview of using sudo su
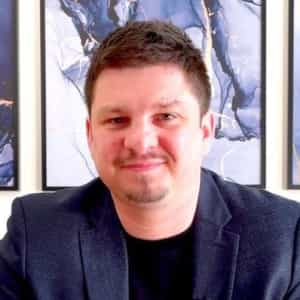
Generate, Sign, and View a CSR With OpenSSL
Learn how to generate, self-sign, and verify certificate signing requests with `openssl`.
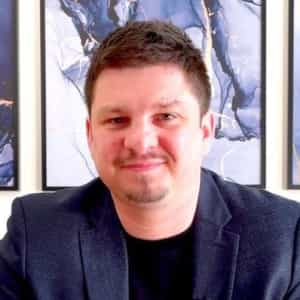
How to use sudo rm -rf safely
We'll help you understand its components
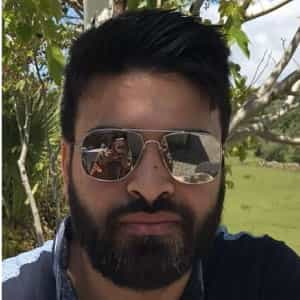
How to run chmod recursively
Using -R is probably not what you want
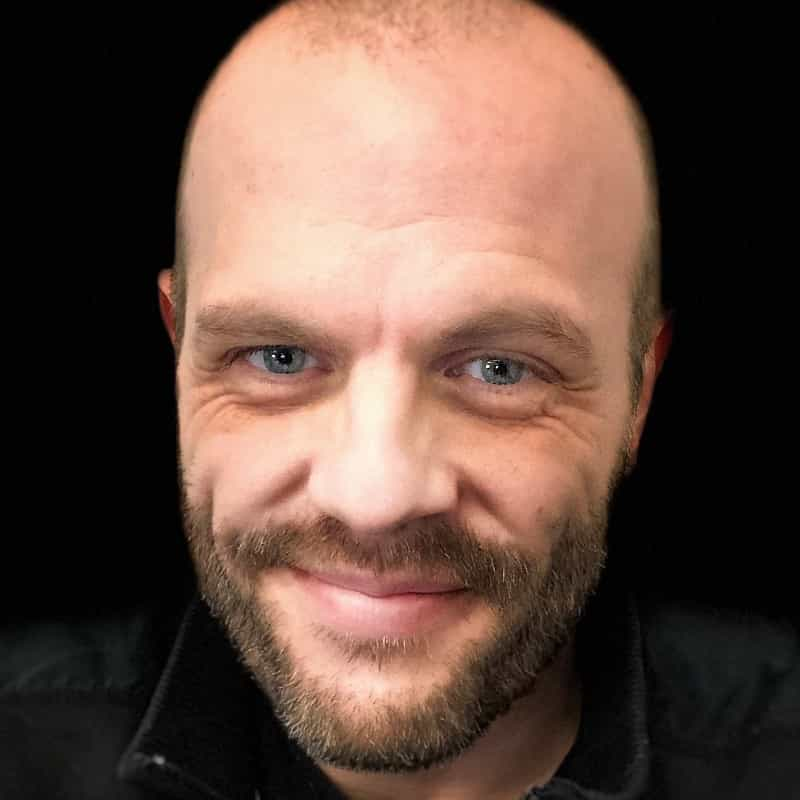
Run Bash Shell In Docker
Start an interactive shell in Docker container
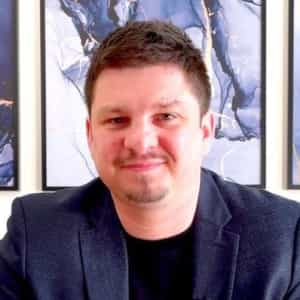
Curl Post Request
Use cURL to send data to a server
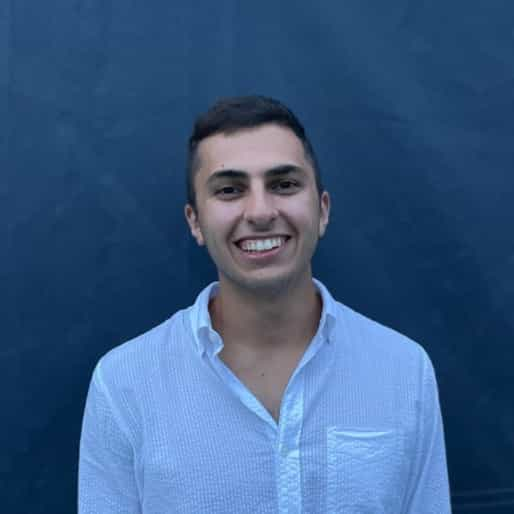
Bash Aliases
Create an alias for common commands
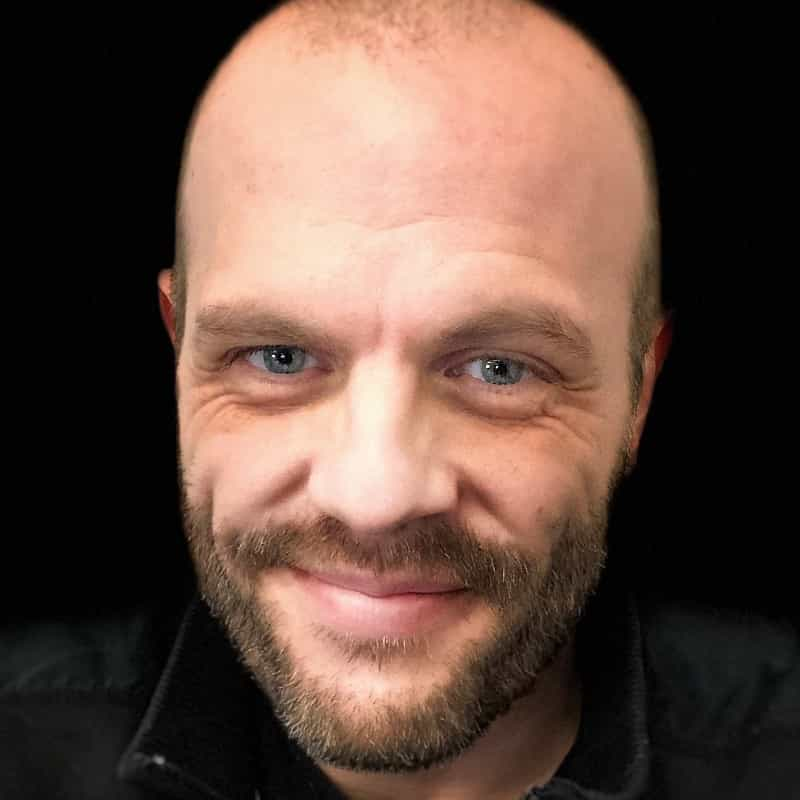